Solved Chisel : Error compiling the sbt component 'compiler-bridge_2.12'
2025-01-09
When running the chisel book example, we got Error compiling the sbt component ‘compiler-bridge_2.12’. Here are the way to solve it
git clone https://github.com/schoeberl/chisel-examples.git
Edit hello-world/build.sbt
scalaVersion := "2.13.12"
scalacOptions ++= Seq(
"-feature",
"-language:reflectiveCalls",
)
// Chisel 3.5
// addCompilerPlugin("edu.berkeley.cs" % "chisel3-plugin" % "6.0.0" cross CrossVersion.full)
// libraryDependencies += "edu.berkeley.cs" %% "chisel3" % "6.0.0"
// libraryDependencies += "edu.berkeley.cs" %% "chiseltest" % "0.6.0"
val chiselVersion = "6.0.0"
addCompilerPlugin("org.chipsalliance" % "chisel-plugin" % chiselVersion cross CrossVersion.full)
libraryDependencies += "org.chipsalliance" %% "chisel" % chiselVersion
libraryDependencies += "edu.berkeley.cs" %% "chiseltest" % "0.6.0" % "test"
Edit hello-world/src/main/scala/Hello.scala
/*
* This code is a minimal hardware described in Chisel.
*
* Blinking LED: the FPGA version of Hello World
*/
import chisel3._
import circt.stage.ChiselStage
/**
* The blinking LED component.
*/
class Hello extends Module {
val io = IO(new Bundle {
val led = Output(UInt(1.W))
})
val CNT_MAX = (50000000 / 2 - 1).U
val cntReg = RegInit(0.U(32.W))
val blkReg = RegInit(0.U(1.W))
cntReg := cntReg + 1.U
when(cntReg === CNT_MAX) {
cntReg := 0.U
blkReg := ~blkReg
}
io.led := blkReg
}
/**
* An object extending App to generate the Verilog code.
*/
object Hello extends App {
println(
ChiselStage.emitSystemVerilog(
new Hello(),
firtoolOpts = Array("-disable-all-randomization", "-strip-debug-info")
)
)
}
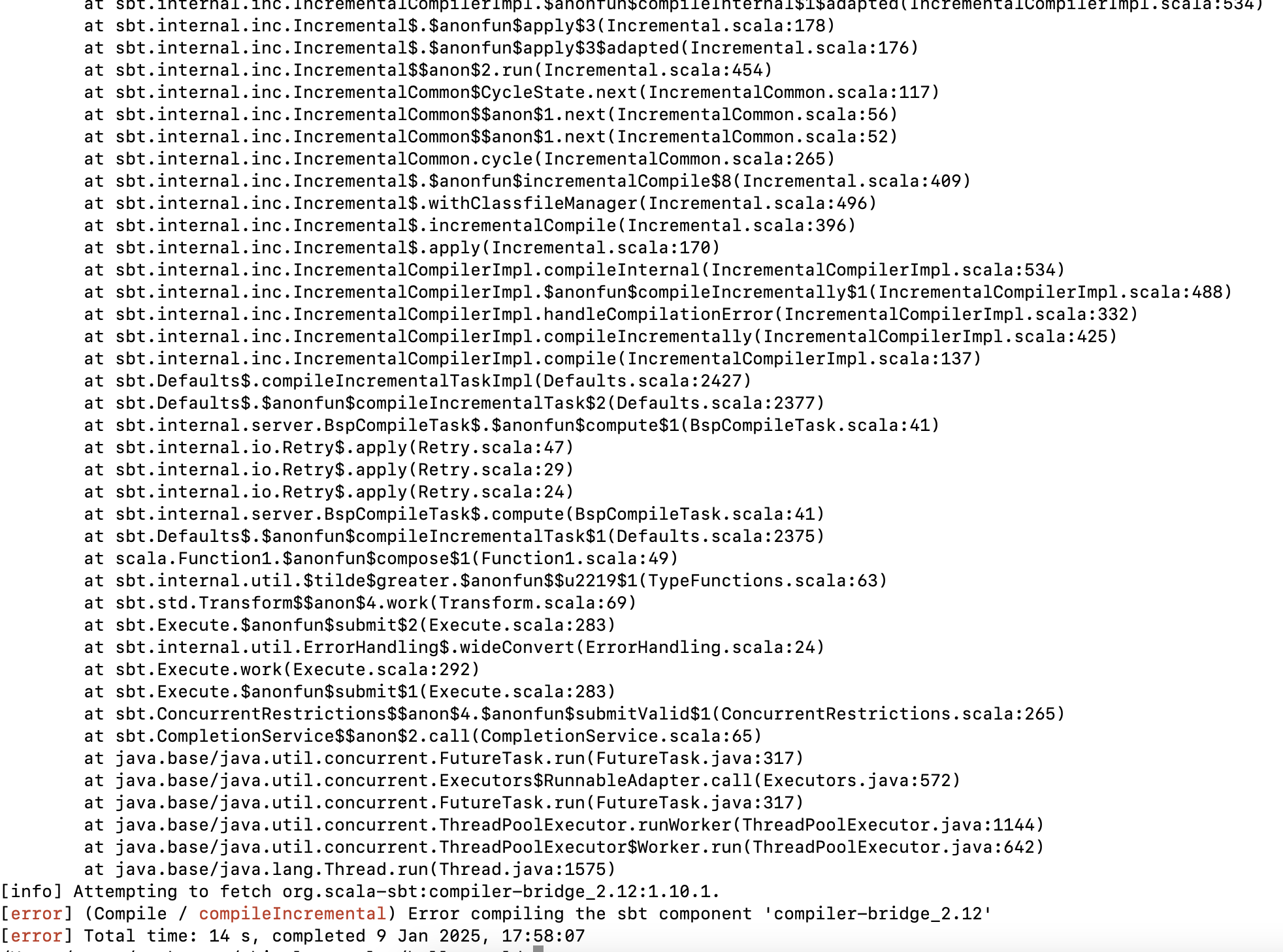