Simplest standalone ApacheDS server
2024-06-09
This code run a standalone ApacheDS server, you can use ApacheDS studio to connect to it, it host localhost:10389
package hk.quantr.apachedsembeddedserver;
import java.io.File;
import java.io.IOException;
import java.util.List;
import org.apache.directory.api.ldap.model.constants.SchemaConstants;
import org.apache.directory.api.ldap.model.schema.LdapComparator;
import org.apache.directory.api.ldap.model.schema.comparators.NormalizingComparator;
import org.apache.directory.api.ldap.model.schema.registries.ComparatorRegistry;
import org.apache.directory.api.ldap.model.schema.registries.SchemaLoader;
import org.apache.directory.api.ldap.schema.extractor.SchemaLdifExtractor;
import org.apache.directory.api.ldap.schema.extractor.impl.DefaultSchemaLdifExtractor;
import org.apache.directory.api.ldap.schema.loader.LdifSchemaLoader;
import org.apache.directory.api.ldap.schema.manager.impl.DefaultSchemaManager;
import org.apache.directory.server.constants.ServerDNConstants;
import org.apache.directory.server.core.DefaultDirectoryService;
import org.apache.directory.server.core.api.InstanceLayout;
import org.apache.directory.server.core.api.partition.Partition;
import org.apache.directory.server.core.api.schema.SchemaPartition;
import org.apache.directory.server.core.factory.JdbmPartitionFactory;
import org.apache.directory.server.core.partition.ldif.LdifPartition;
import org.apache.directory.server.ldap.LdapServer;
import org.apache.directory.server.protocol.shared.transport.TcpTransport;
import org.apache.directory.server.protocol.shared.transport.Transport;
/**
*
* @author Peter <[email protected]>
*/
public class ApacheDSEmbeddedServer {
public static void main(String[] args) throws ClassNotFoundException, Exception {
DefaultDirectoryService directoryService = new DefaultDirectoryService();
File workingDirector = new File("working_dir");
InstanceLayout instanceLayout = new InstanceLayout(workingDirector);
directoryService.setInstanceLayout(instanceLayout);
File schemaRepository = new File(workingDirector.getName(), "schema");
SchemaLdifExtractor extractor = new DefaultSchemaLdifExtractor(workingDirector);
try {
extractor.extractOrCopy();
} catch (IOException ioe) {
// The schema has already been extracted, bypass
}
SchemaLoader loader = new LdifSchemaLoader(schemaRepository);
DefaultSchemaManager schemaManager = new DefaultSchemaManager(loader);
schemaManager.loadAllEnabled();
ComparatorRegistry comparatorRegistry = schemaManager.getComparatorRegistry();
for (LdapComparator<?> comparator : comparatorRegistry) {
if (comparator instanceof NormalizingComparator) {
((NormalizingComparator) comparator).setOnServer();
}
}
directoryService.setSchemaManager(schemaManager);
// Init the schema partation
LdifPartition ldifPartition = new LdifPartition(schemaManager, directoryService.getDnFactory());
ldifPartition.setPartitionPath(new File(workingDirector.getName(), "schema").toURI());
SchemaPartition schemaPartition = new SchemaPartition(schemaManager);
schemaPartition.setWrappedPartition(ldifPartition);
directoryService.setSchemaPartition(schemaPartition);
List<Throwable> errors = schemaManager.getErrors();
// Inject the System Partition
JdbmPartitionFactory partitionFactory = new JdbmPartitionFactory();
Partition systemPartition = partitionFactory.createPartition(directoryService.getSchemaManager(), directoryService.getDnFactory(), "system", ServerDNConstants.SYSTEM_DN, 500, new File(directoryService.getInstanceLayout().getPartitionsDirectory(), "system"));
systemPartition.setSchemaManager(directoryService.getSchemaManager());
partitionFactory.addIndex(systemPartition, SchemaConstants.OBJECT_CLASS_AT, 100);
directoryService.setSystemPartition(systemPartition);
// schemaManager.loadAllEnabled();
// SchemaPartition schemaPartition = new SchemaPartition(schemaManager);
// directoryService.setSchemaPartition(schemaPartition);
LdapServer ldapServer = new LdapServer();
ldapServer.setDirectoryService(directoryService);
ldapServer.setServiceName("DefaultLDAP");
Transport ldap = new TcpTransport("0.0.0.0", 10389, 3, 5);
ldapServer.addTransports(ldap);
// LdifFileLoader ldifLoader = new LdifFileLoader(directoryService.getAdminSession(), "users.ldif");
// ldifLoader.execute();
directoryService.startup();
ldapServer.start();
}
}
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>hk.quantr</groupId>
<artifactId>ApacheDSEmbeddedServer</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>ApacheDS Embedded Server</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>21</maven.compiler.source>
<maven.compiler.target>21</maven.compiler.target>
<exec.mainClass>hk.quantr.apachedsembeddedserver.ApacheDSEmbeddedServer</exec.mainClass>
</properties>
<dependencies>
<dependency>
<groupId>org.apache.directory.server</groupId>
<artifactId>apacheds-all</artifactId>
<version>2.0.0.AM27</version>
</dependency>
</dependencies>
</project>
Then you can connect it by ApacheDS Studio
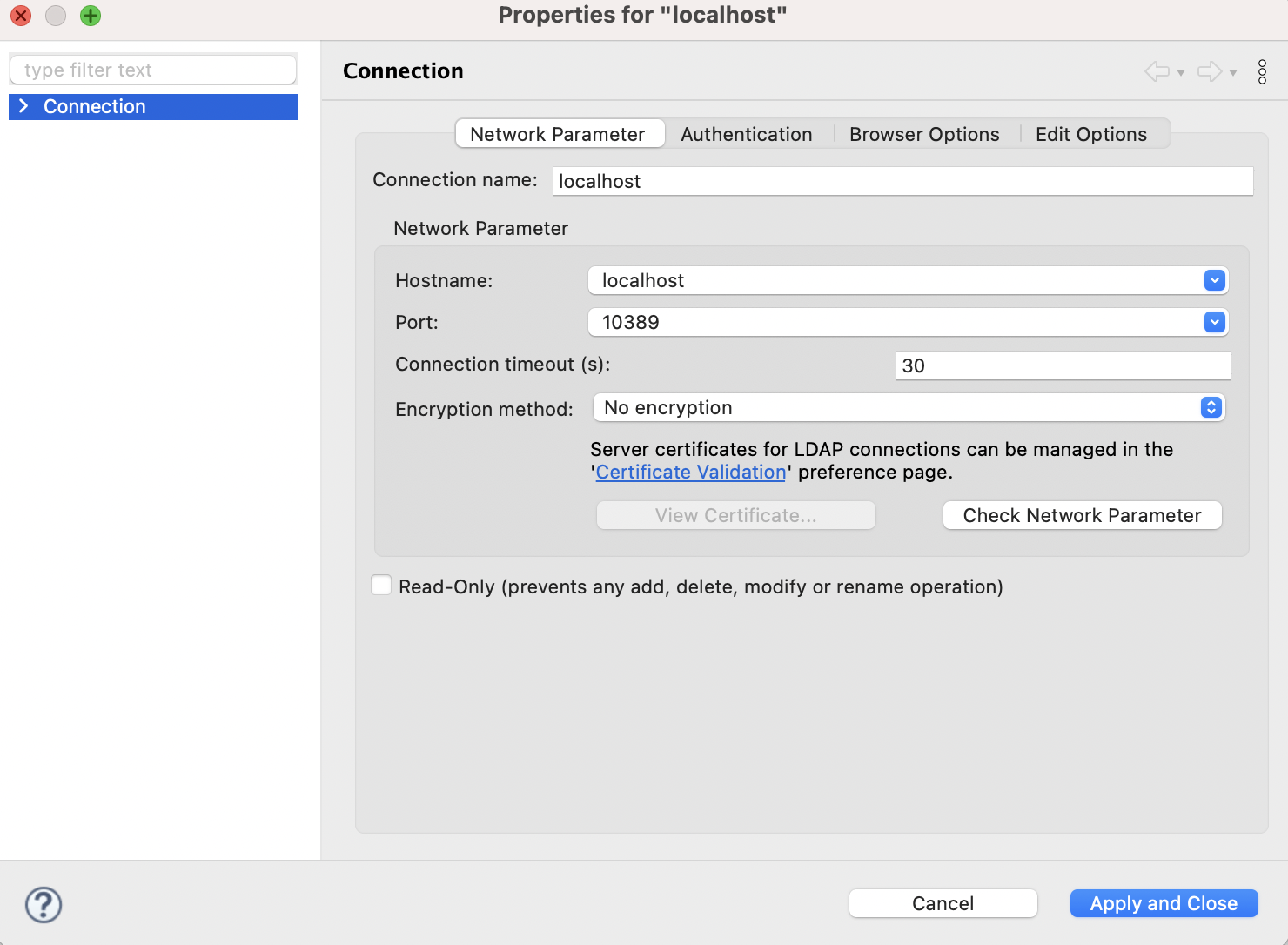
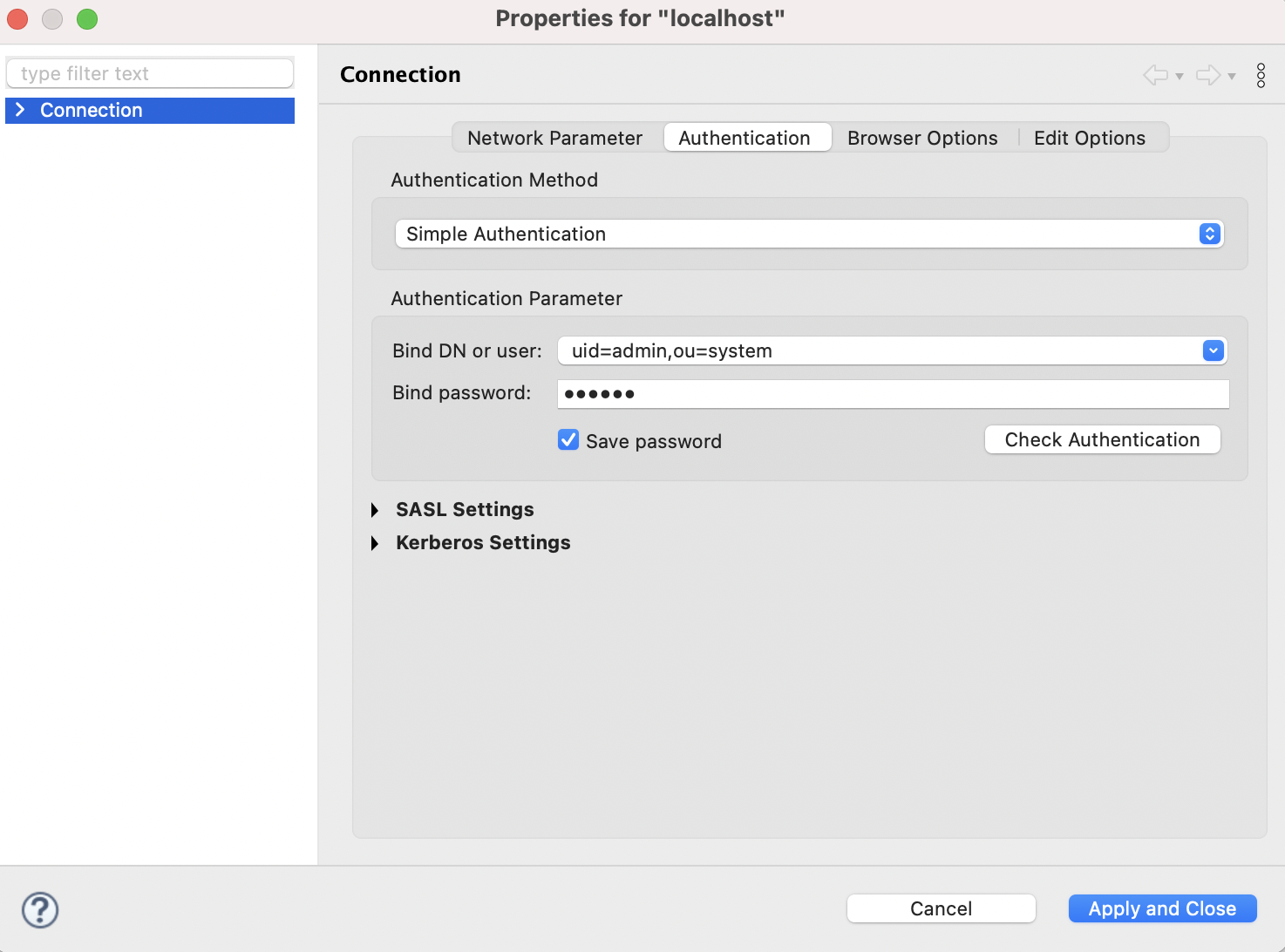