import chisel3._
import chisel3.util._
import _root_.circt.stage.ChiselStage
class MyRam extends Module {
val io = IO(new Bundle {
val addr = Input(UInt(8.W))
val dataIn = Input(UInt(8.W))
val dataOut = Output(UInt(8.W))
val write = Input(Bool())
})
val symcMem = SyncReadMem(256, UInt(8.W))
val initDone = RegInit(false.B)
when(!initDone) {
io.dataOut := 0.U
initDone := true.B
}.otherwise {
io.dataOut := DontCare // Ensure dataOut is not driven by default
when(io.write) {
symcMem.write(io.addr, io.dataIn)
}.otherwise {
io.dataOut := symcMem.read(io.addr)
}
}
}
object MyMain extends App {
println(
ChiselStage.emitSystemVerilog(
gen = new MyRam,
firtoolOpts = Array("-disable-all-randomization")
)
)
}
import chisel3._
import chisel3.util._
import _root_.circt.stage.ChiselStage
class MyRam extends Module {
val io = IO(new Bundle {
val addr = Input(UInt(8.W))
val dataIn = Input(UInt(8.W))
val dataOut = Output(UInt(8.W))
val write = Input(Bool())
})
io.dataOut := DontCare
val symcMem = SyncReadMem(256, UInt(8.W))
when(io.write) {
symcMem.write(io.addr, io.dataIn)
}.otherwise {
io.dataOut := symcMem.read(io.addr)
}
}
object MyMain extends App {
println(
ChiselStage.emitSystemVerilog(
gen = new MyRam,
firtoolOpts = Array("-disable-all-randomization")
)
)
}
if you want to send one single byte
this is not working
writer.write(new Int32Array([0x4]).buffer);
this work
writer.write(new Uint8Array([0x4]));
There are two mode in micropython: REPL vs paste mode. I guess the uart of my ESP32 board has no flow control, so you can’t send all bytes at once, because it is too fast, so I sleep 100 ms for every 100 bytes.
REPL mode
You can’t just send your python code, you need to remind two things: the indent and the return of indent. See the below diagram, in line 26 should be one indent but you can’t have it, because the line 25 is indent-ed so all next lines are supposed no need to indent again. So you can see from line 26 to 32, there is no indent. Secondly, in line 34, you need \r to return from indent to no-indent

Paste mode
In paste mode, you DON’T have to change anything to your code like the last section. But you need to send 0x5 to enter paste mode and send 0x4 to leave paste mode, after that, the program will run right after it. See line 61 and 72
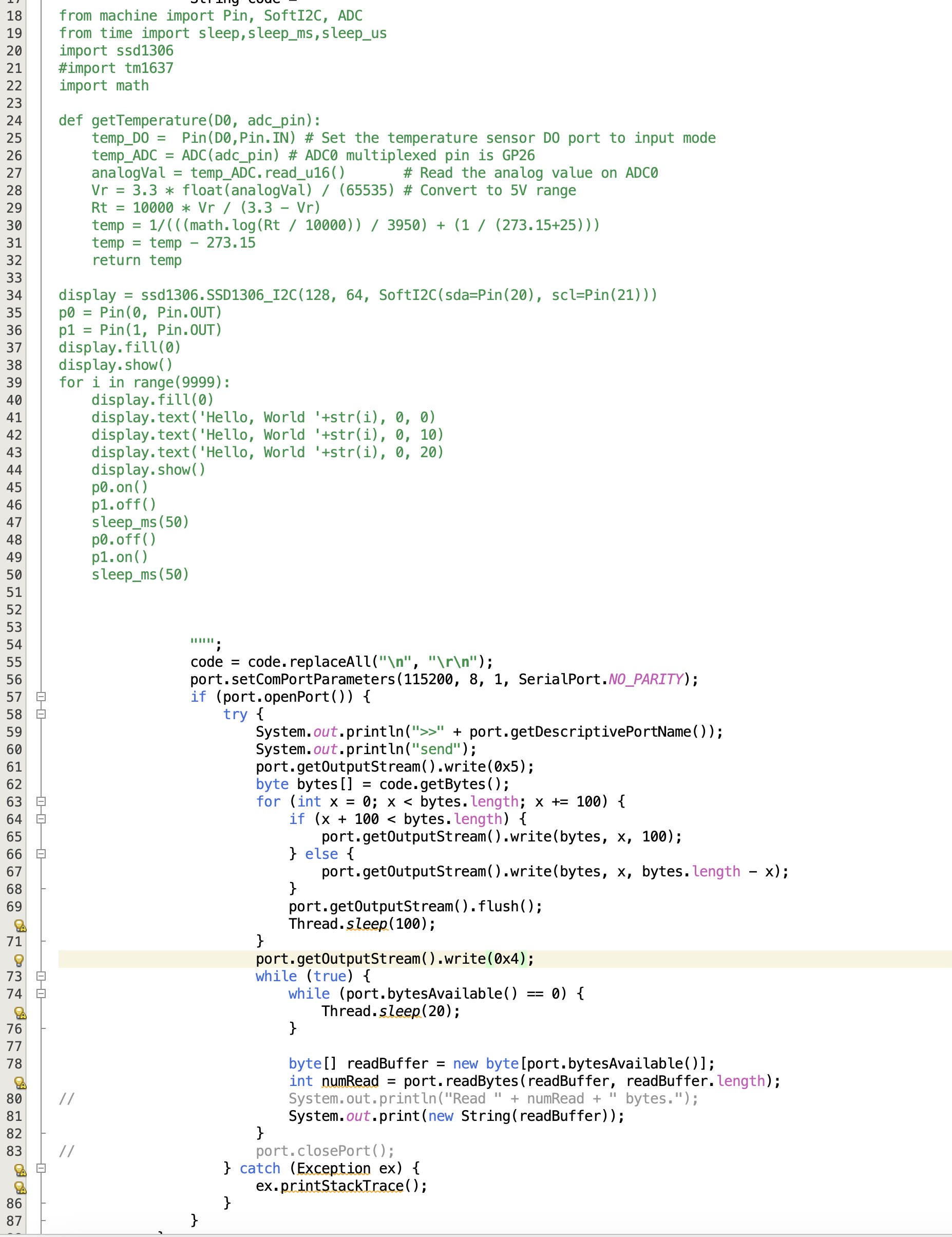
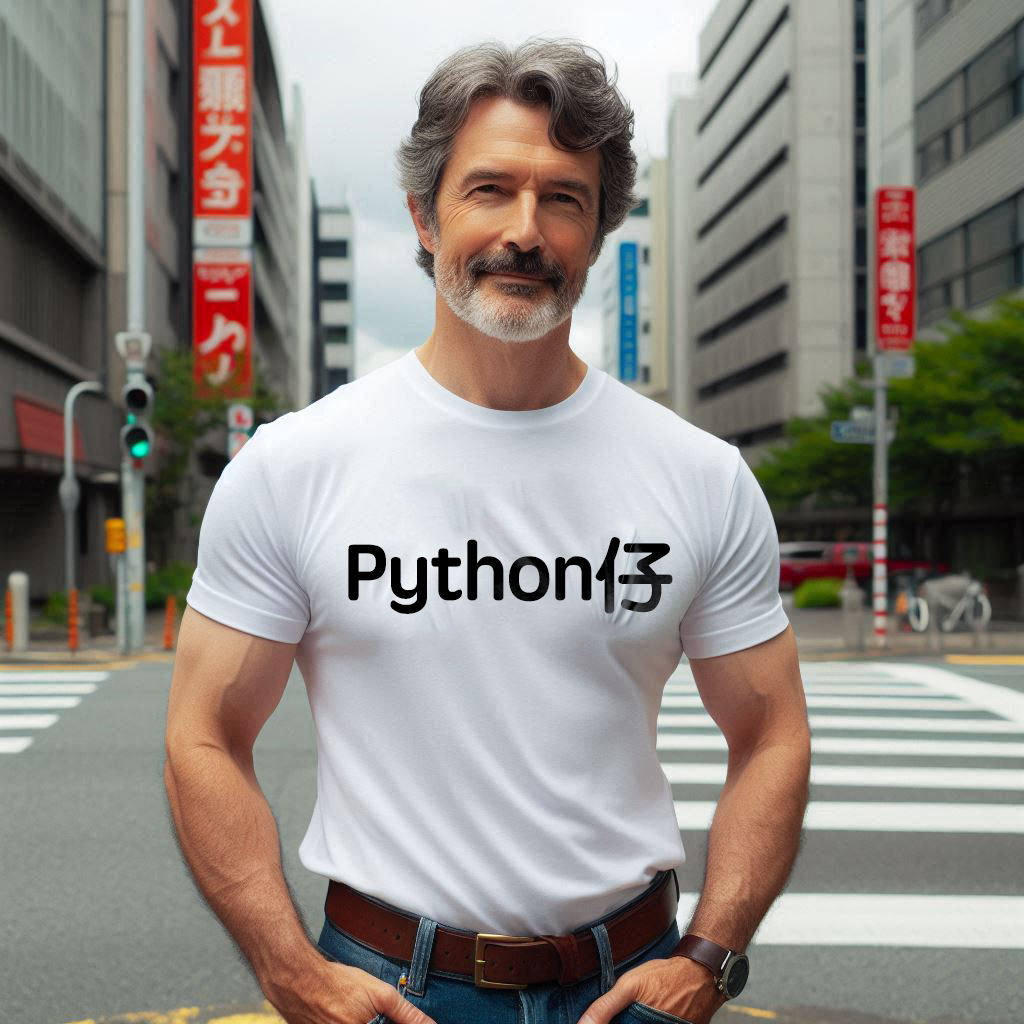
香港有人係咁,廿幾歲畢業出黎做野,做左幾年對隻language開始熟,佢地唔係鑽落去language下面睇下發生緊乜野,例如啲code點compile呀,debug點做呀咁。佢地會作一出個難以解釋嘅現象,就係跳去另一隻high level language到再玩過,而當佢地玩到興起玩左幾年,佢地再次唔係想知道隻language嘅底層,而係再一次又跳去其它high level language到碌多一次,難道唔想知揸係手裏面把刀係咩黎?我叫呢一種現象做「香港Python仔現象」。舉個例子,2003年班友玩vc++,跟住跳去c#,跟住又跳去java,而家又跳去python。因為咁樣跳法本質上對過去學嘅語言都唔方認識得深,所以如果而家有個阿叔好推崇python,對其它language只停留係誇誇其談攞唔出到戰鬥力,佢大概率係咁。
要改變,就要做好科普,揭露python底層所有野,咁先至有機會改變。但呢班友會唔會變未知。
When running the chisel book example, we got Error compiling the sbt component ‘compiler-bridge_2.12’. Here are the way to solve it
git clone https://github.com/schoeberl/chisel-examples.git
Edit hello-world/build.sbt
scalaVersion := "2.13.12"
scalacOptions ++= Seq(
"-feature",
"-language:reflectiveCalls",
)
// Chisel 3.5
// addCompilerPlugin("edu.berkeley.cs" % "chisel3-plugin" % "6.0.0" cross CrossVersion.full)
// libraryDependencies += "edu.berkeley.cs" %% "chisel3" % "6.0.0"
// libraryDependencies += "edu.berkeley.cs" %% "chiseltest" % "0.6.0"
val chiselVersion = "6.0.0"
addCompilerPlugin("org.chipsalliance" % "chisel-plugin" % chiselVersion cross CrossVersion.full)
libraryDependencies += "org.chipsalliance" %% "chisel" % chiselVersion
libraryDependencies += "edu.berkeley.cs" %% "chiseltest" % "0.6.0" % "test"
Edit hello-world/src/main/scala/Hello.scala
/*
* This code is a minimal hardware described in Chisel.
*
* Blinking LED: the FPGA version of Hello World
*/
import chisel3._
import circt.stage.ChiselStage
/**
* The blinking LED component.
*/
class Hello extends Module {
val io = IO(new Bundle {
val led = Output(UInt(1.W))
})
val CNT_MAX = (50000000 / 2 - 1).U
val cntReg = RegInit(0.U(32.W))
val blkReg = RegInit(0.U(1.W))
cntReg := cntReg + 1.U
when(cntReg === CNT_MAX) {
cntReg := 0.U
blkReg := ~blkReg
}
io.led := blkReg
}
/**
* An object extending App to generate the Verilog code.
*/
object Hello extends App {
println(
ChiselStage.emitSystemVerilog(
new Hello(),
firtoolOpts = Array("-disable-all-randomization", "-strip-debug-info")
)
)
}
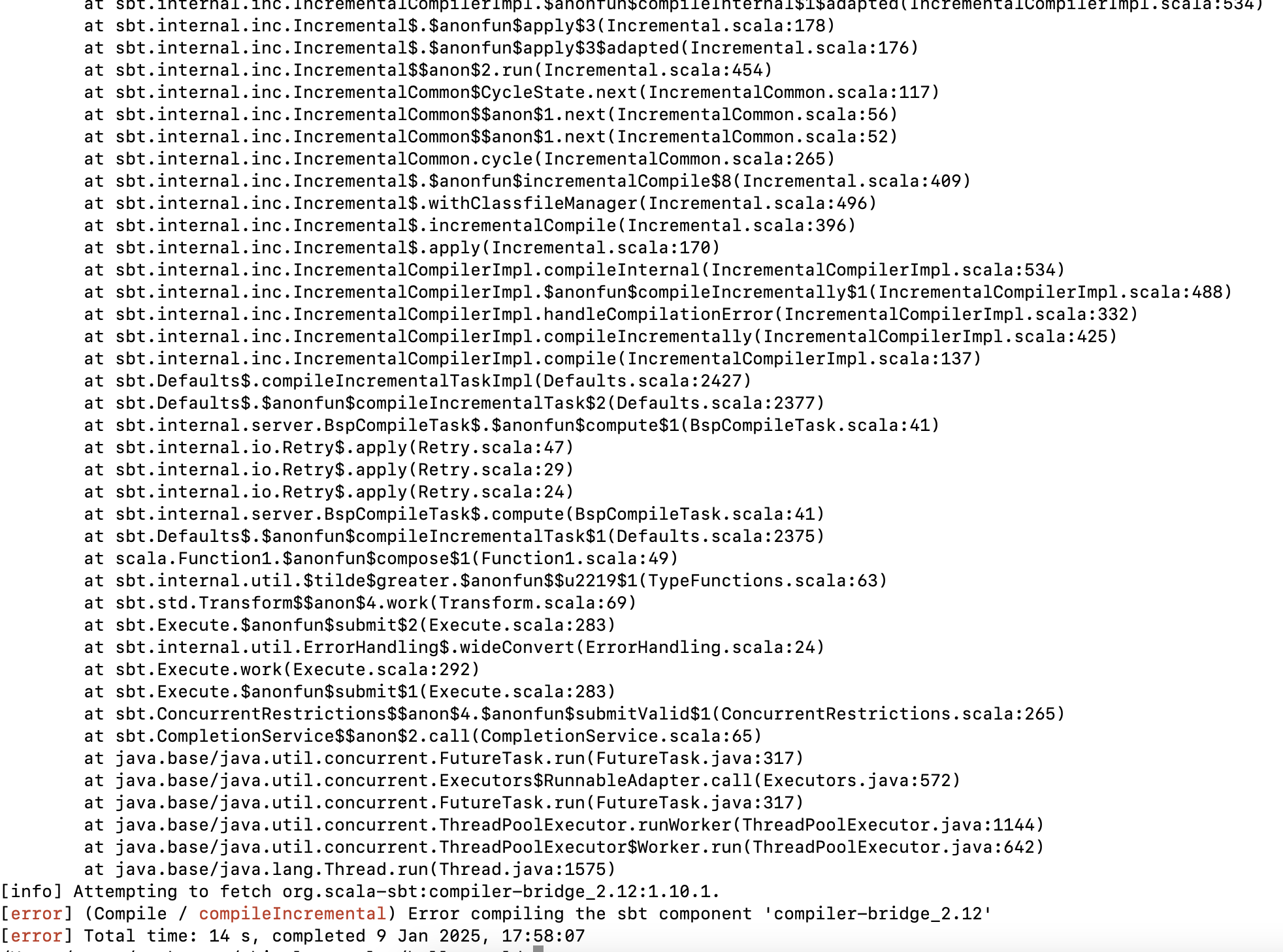
香港人嘅老土活該沒有科技,我而家分析俾你地睇香港人嘅老土係有幾老土,以及老土嘅香港人點解覺得自己唔老土
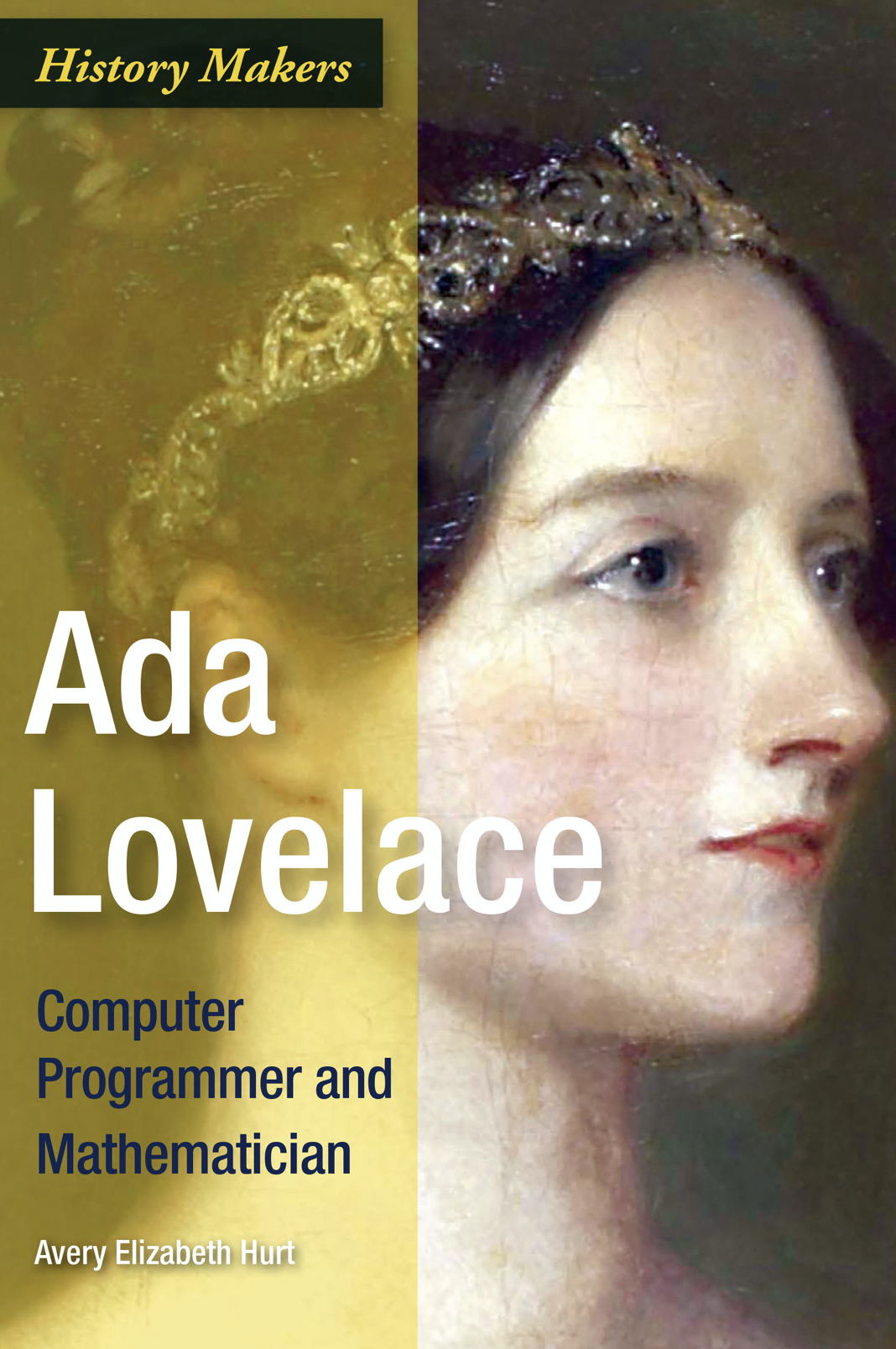
第一老土 : 你憑咩
佢地所謂嘅論證不外乎係黎自你所受嘅教育同埋經驗,佢地會話你又唔係哈佛MIT學咩野人搞之類嘅言論,但呢班無讀歷史嘅人唔知好多科學家都係自學為主。(見下圖)
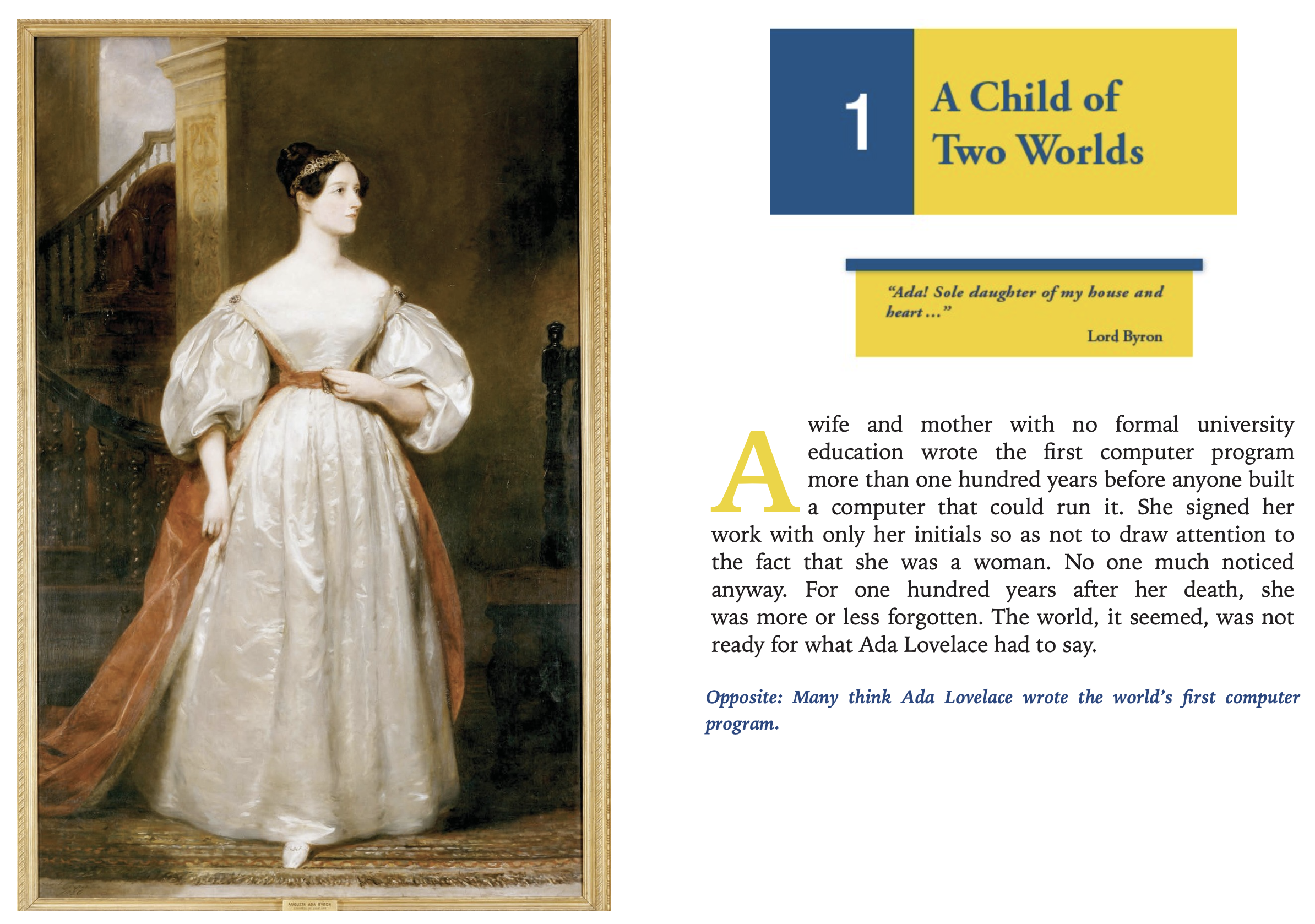
香港人老土在永遠人地努力做,佢地就會問”你憑咩”,其實呢一句好無敵,因為當你做緊而又未做完嘅時間你的確無力反駁呢一句,由其是你係做緊一啲你自己都唔知做唔做到嘅野時,你更加無力反駁。但呢班友永遠唔明,科學嘅發現好多時就係意外發現,好多野唔做就唔唔知得唔得,而呢班咁嘅人最叻就係用呢句去質疑你,佢地嘅內心世界就係「嘩,你做呀,你有無諗過㗎」,跟住就會覺得自己好似好有經驗,好似真係以為自己曾經做過咁,知道你條路係唔通,其實個現實就係,呢班友根本無做過,而更深一層嘅就係,佢地連去嘗試嘅基本實力都無,佢地唔係連個for-loop都寫唔出就係技術只夠寫啲script仔,真係可憐。
第二老土 : 話人畫餅
呢個世界唔係所有人都需要理想,而理想呢樣野個實現率必定為低。香港地當然多人畫餅,但係係唔係畫餅好容易分,得個噏字唔做咪就係畫餅囉,會做嘅又點會算係畫餅呢,但係呢班咁嘅人就最叻攻擊啲為理想而行動嘅人。佢地個底其實係數佬,內心世界非常現實,我估計佢地後生嘅時候都曾經有理想,但手料跟唔上人到中年完全無哂動手能力,思想上變得越黎越現實,腦裏面諗一百個可能性去否定執行嘅成功率,不停俾借口自己逃避執行以至完全喪失執行力,佢地係編程上嘅實力只能點評人地寫嘅program,而自己乜都寫唔到。
Ada係連電都未係好識運用嘅年代就話要令機器有計算能力,如果香港班友返去佢嗰個時代,呢班友就會話人畫餅。嗰個時代根本就係非常簡陋。當呢班友話人畫餅,佢地嘅內心有一種好充盈嘅感覺,佢地會覺得:「嘿嘿,我真係叻,無俾你利用到」。但係個現實係咩呢,就係呢班友手料根本連俾人利用嘅資格都無,同你講下理想只係發下噏瘋而矣,唔好諗得自己咁有料可以俾人利用。
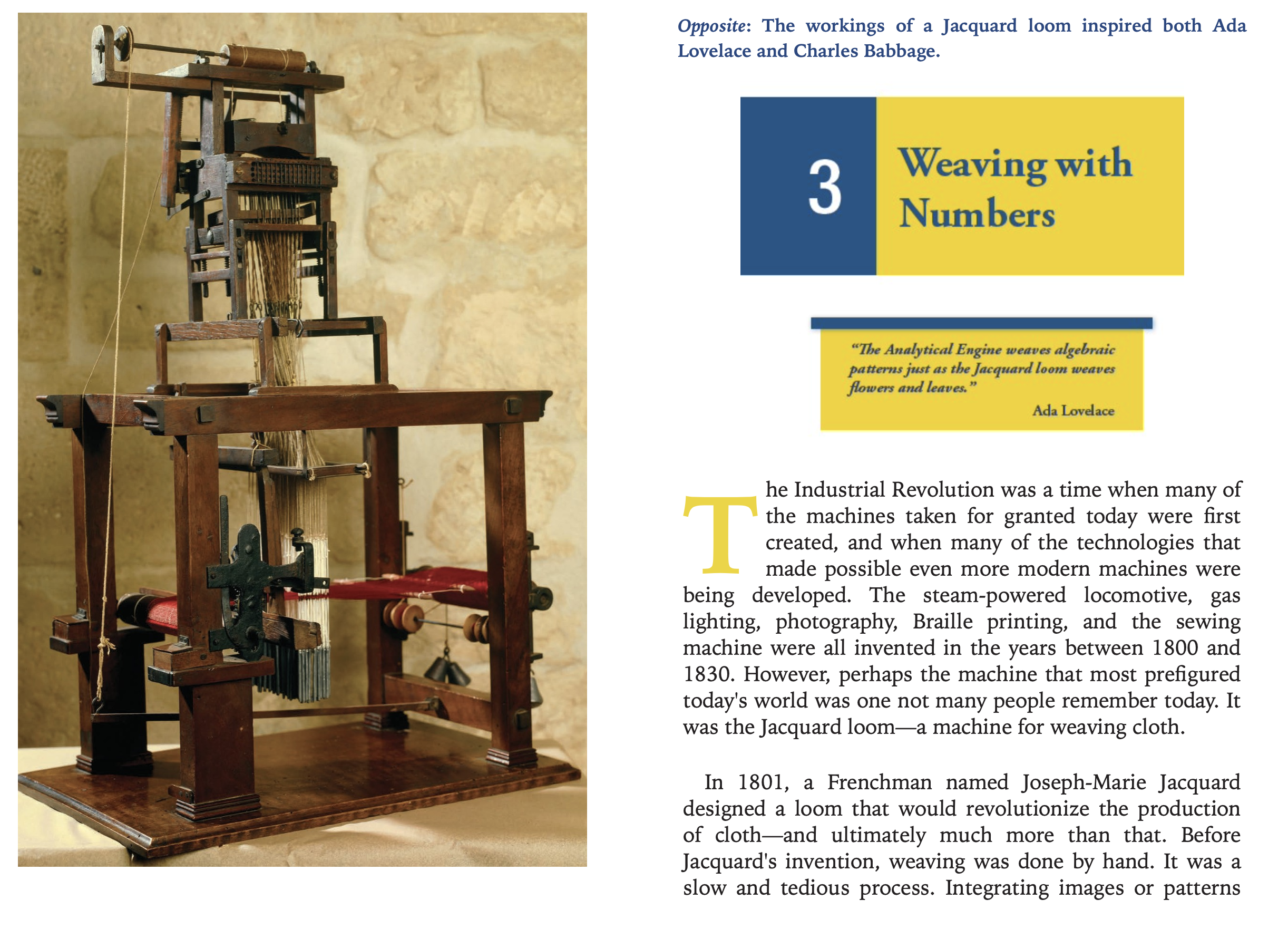
第三老土:話做唔做或者揾借口唔繼續做
話做唔做嘅人主要會話你唔夠條件做,做緊又揾借口唔繼續做嘅人會話發現你原來唔夠料做,又或者話發現你身上有啲缺點而唔再同你合作做。總之無論係咩原因都好,結果就係唔做。我地睇返Ada Lovelace個Case,當佢話要做一部Thinking machine嘅時候,佢mentor Charles Babbage唔會因為佢太天馬行空而屈佢畫餅,想反地會動手一齊做。而Charles Babbage亦都唔會話Ada無足夠嘅錢同埋未讀過大學就話人無料而做做下唔做。
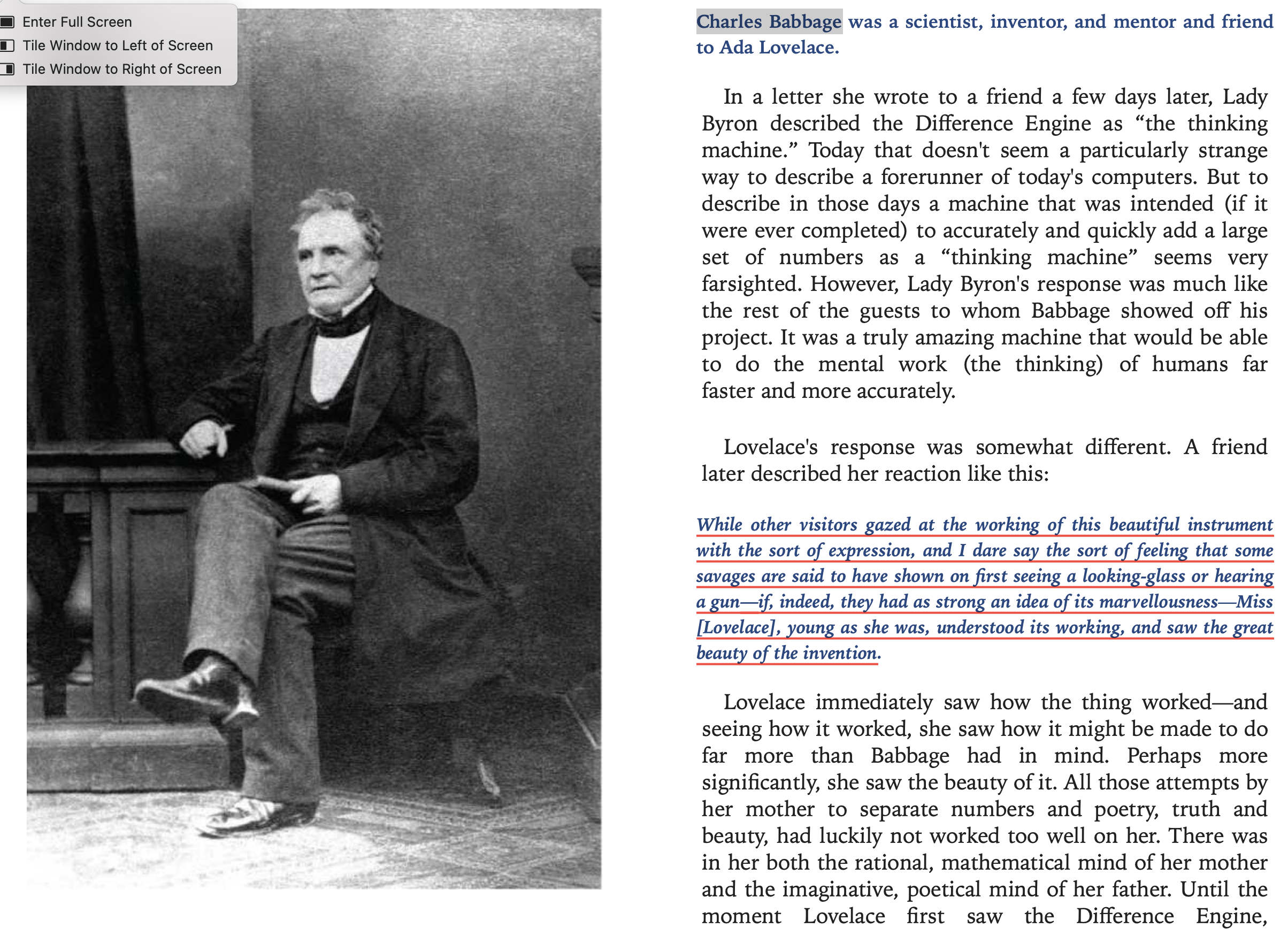
https://conorfennell.github.io/scala-zen/articles/sbt.html
sbt "tasks -v"
bgRun Start an application's default main class as a background job
bgRunMain Start a provided main class as a background job
clean Deletes files produced by the build, such as generated sources, compiled classes, and task caches.
compile Compiles sources.
console Starts the Scala interpreter with the project classes on the classpath.
consoleProject Starts the Scala interpreter with the sbt and the build definition on the classpath and useful imports.
consoleQuick Starts the Scala interpreter with the project dependencies on the classpath.
copyResources Copies resources to the output directory.
deliver Generates the Ivy file for publishing to a repository.
deliverLocal Generates the Ivy file for publishing to the local repository.
dependencyClasspath The classpath consisting of internal and external, managed and unmanaged dependencies.
dependencyClasspathAsJars The classpath consisting of internal and external, managed and unmanaged dependencies, all as JARs.
doc Generates API documentation.
exportedProductJars Build products that go on the exported classpath as JARs.
exportedProductJarsIfMissing Build products that go on the exported classpath as JARs if missing.
exportedProductJarsNoTracking Just the exported classpath as JARs without triggering the compilation.
fullClasspath The exported classpath, consisting of build products and unmanaged and managed, internal and external dependencies.
fullClasspathAsJars The exported classpath, consisting of build products and unmanaged and managed, internal and external dependencies, all as JARs.
internalDependencyAsJars The internal (inter-project) classpath as JARs.
javaOptions Options passed to a new JVM when forking.
javacOptions Options for the Java compiler.
mainClass Defines the main class for packaging or running.
makePom Generates a pom for publishing when publishing Maven-style.
manipulateBytecode Manipulates generated bytecode
mappings Defines the mappings from a file to a path, used by packaging, for example.
package Produces the main artifact, such as a binary jar. This is typically an alias for the task that actually does the packaging.
packageBin Produces a main artifact, such as a binary jar.
packageDoc Produces a documentation artifact, such as a jar containing API documentation.
packageSrc Produces a source artifact, such as a jar containing sources and resources.
printWarnings Shows warnings from compilation, including ones that weren't printed initially.
publish Publishes artifacts to a repository.
publishLocal Publishes artifacts to the local Ivy repository.
publishM2 Publishes artifacts to the local Maven repository.
publishTo The resolver to publish to.
publisher Provides the sbt interface to publisher
run Runs a main class, passing along arguments provided on the command line.
runMain Runs the main class selected by the first argument, passing the remaining arguments to the main method.
scalacOptions Options for the Scala compiler.
test Executes all tests.
testOnly Executes the tests provided as arguments or all tests if no arguments are provided.
testOptions Options for running tests.
testQuick Executes the tests that either failed before, were not run or whose transitive dependencies changed, among those provided as arguments.
unmanagedClasspath Classpath entries (deep) that are manually managed.
unmanagedJars Classpath entries for the current project (shallow) that are manually managed.
unmanagedResources Unmanaged resources, which are manually created.
unmanagedSources Unmanaged sources, which are manually created.
update Resolves and optionally retrieves dependencies, producing a report.
updateClassifiers Resolves and optionally retrieves classified artifacts, such as javadocs and sources, for dependency definitions, transitively.
updateSbtClassifiers Resolves and optionally retrieves classifiers, such as javadocs and sources, for sbt, transitively.
woo Woo
MX512G : 低压单通道有刷直流电机驱动器, RMB $2
MX1616S : 双路有刷直流马达驱动电路, RMB $7.8
TRSP5040A : SOP8語音OTP芯片40秒碩呈語音播報IC程序開發, RMB 0.27
RF2520A : 无线遥控收发器芯片, RMB $4.3
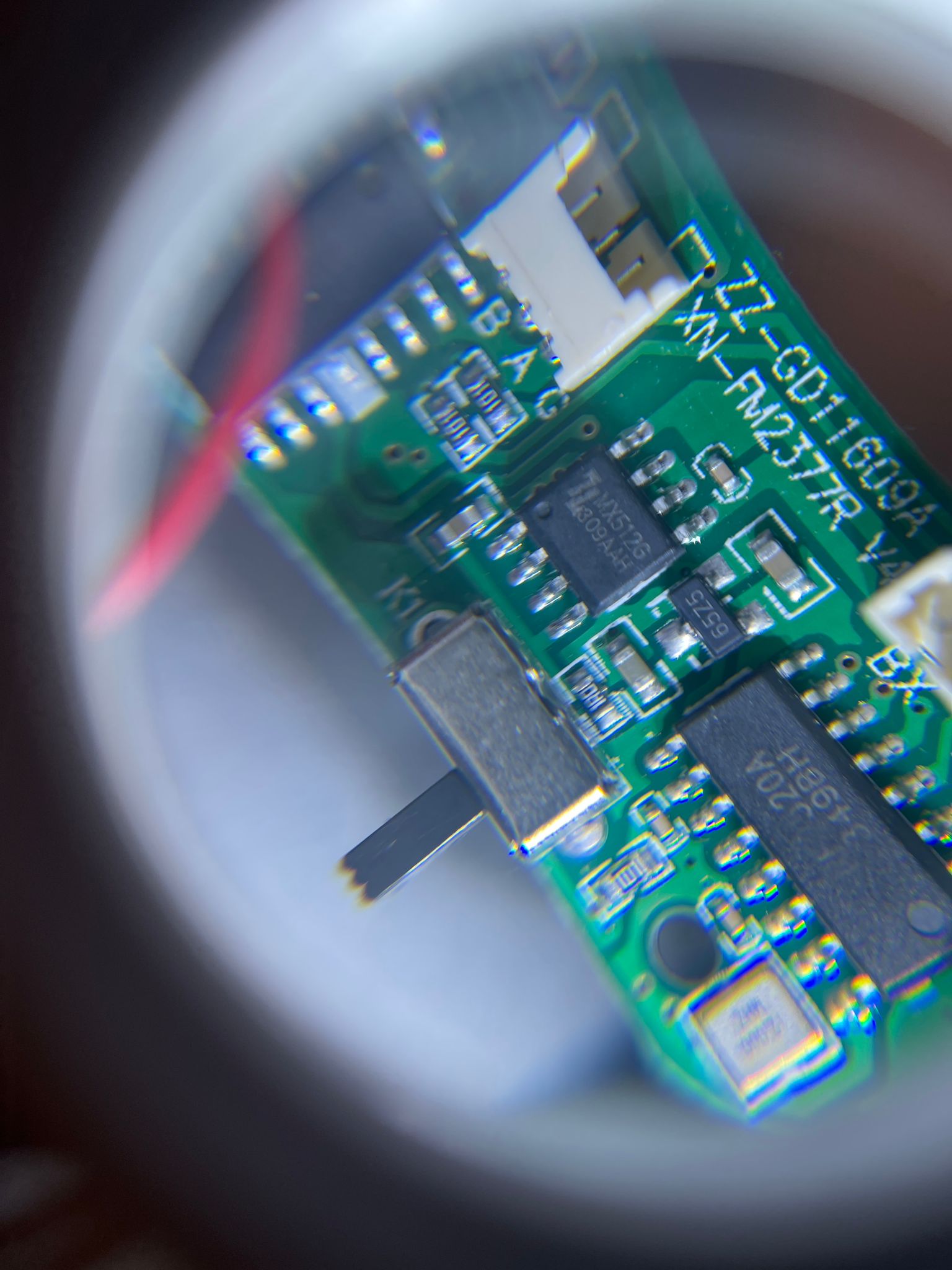
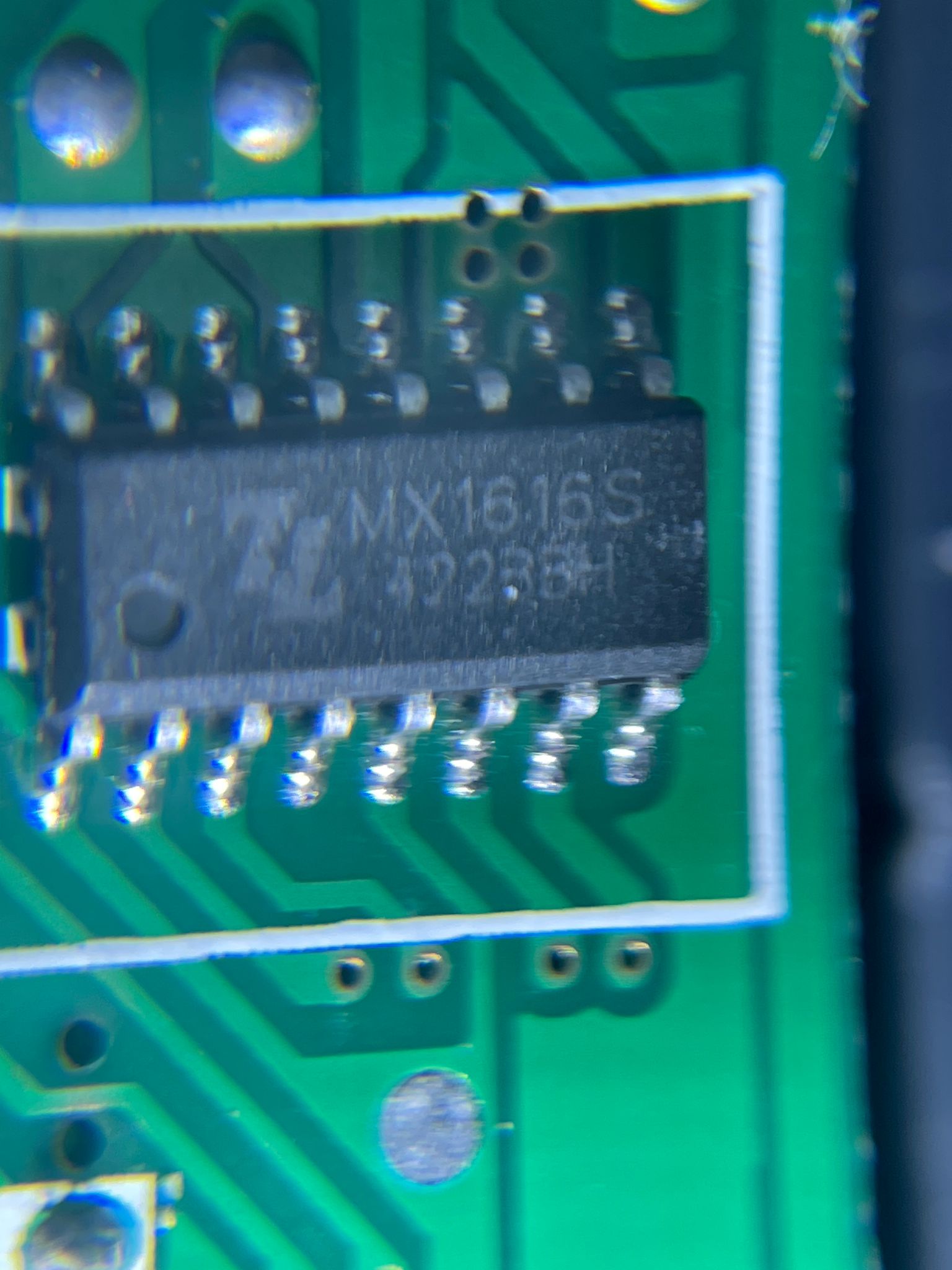
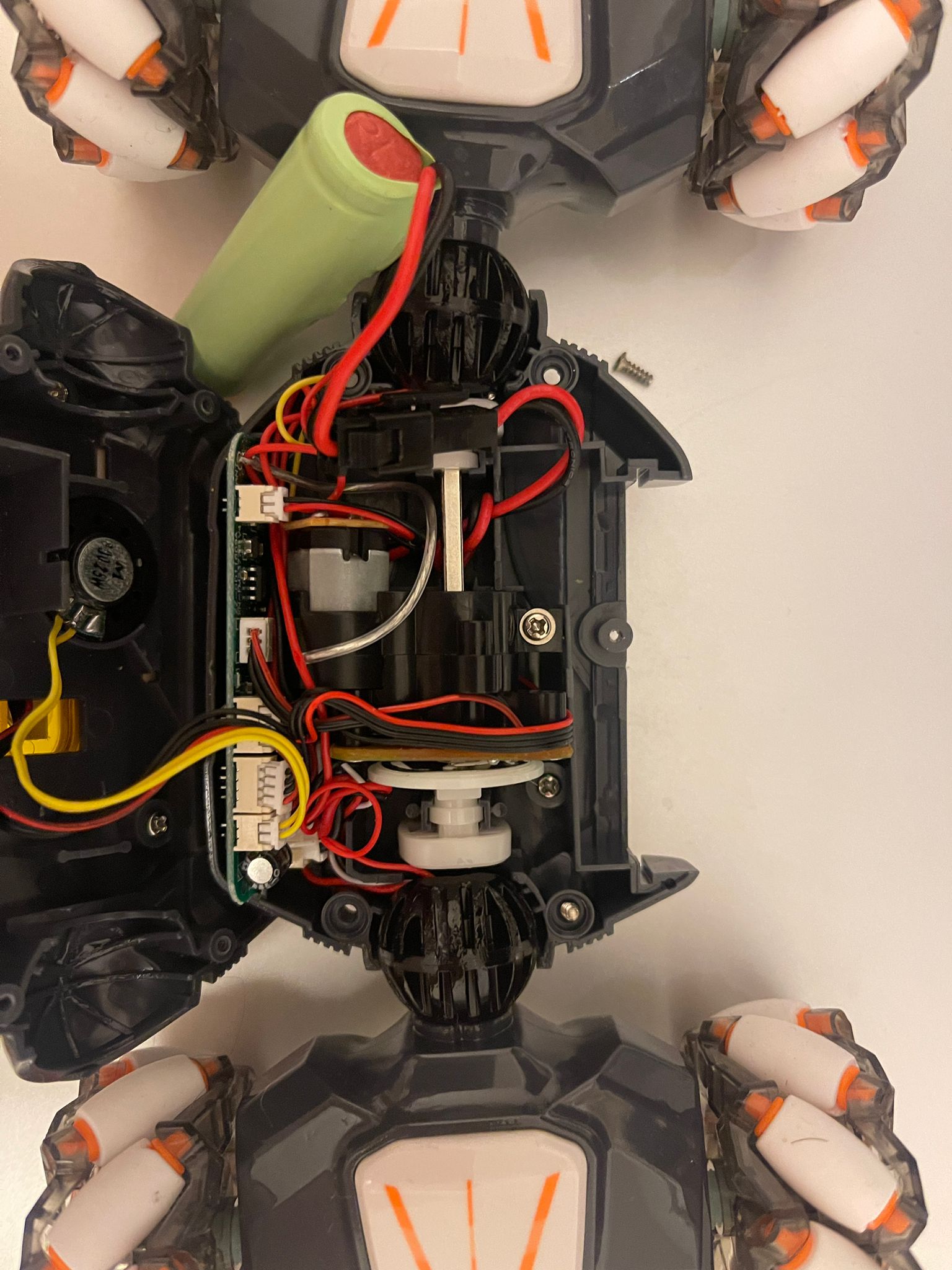
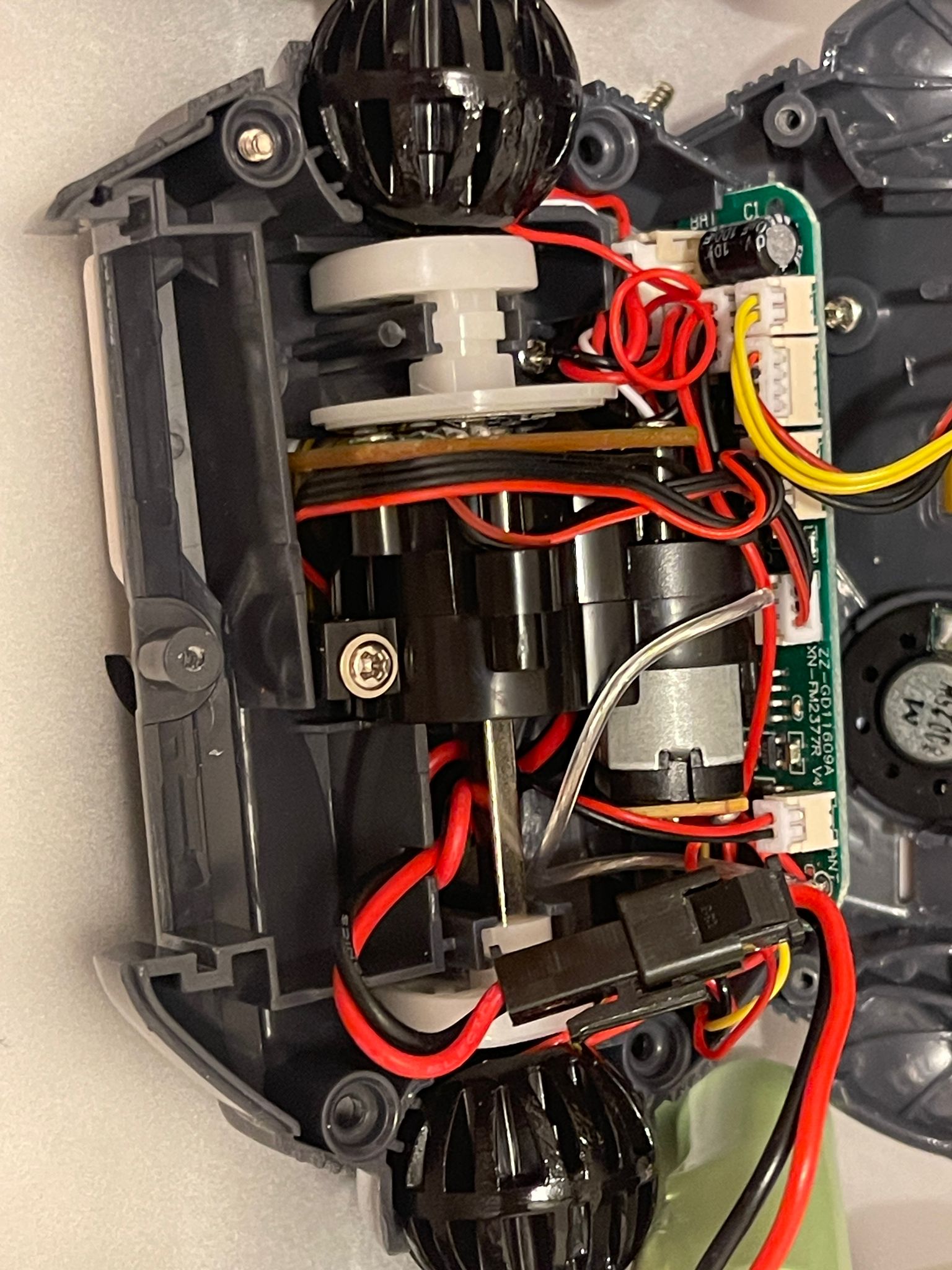
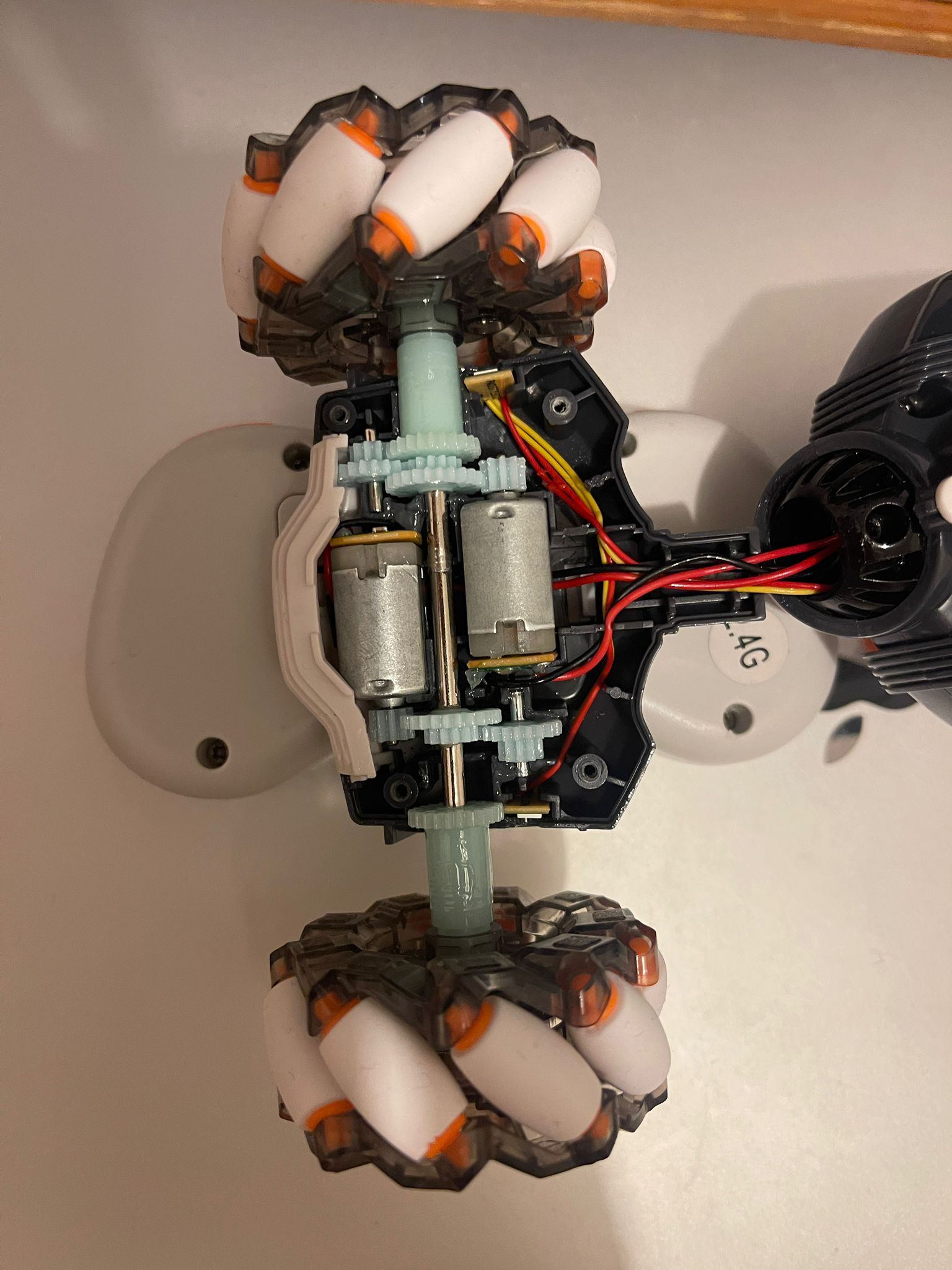
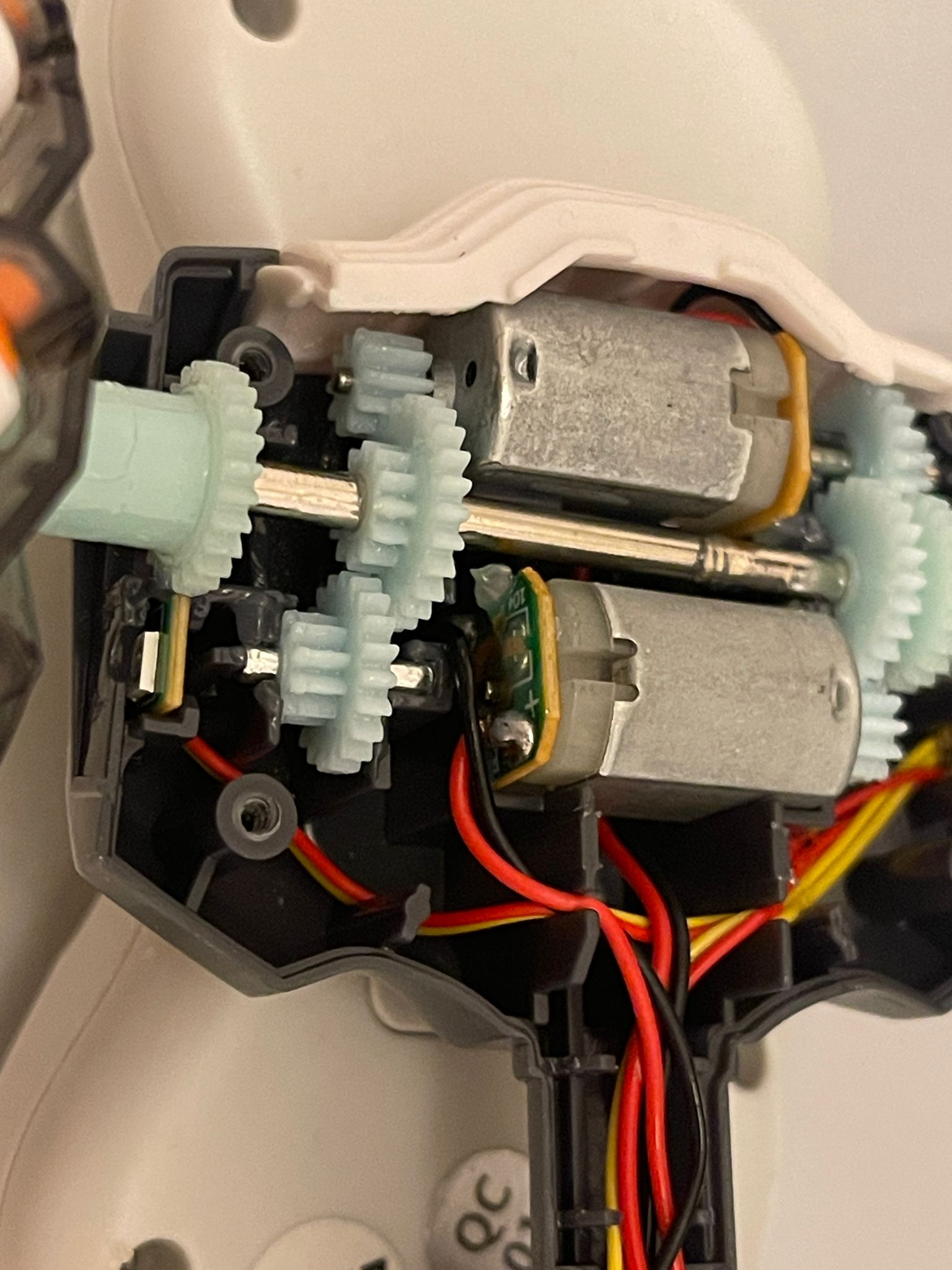
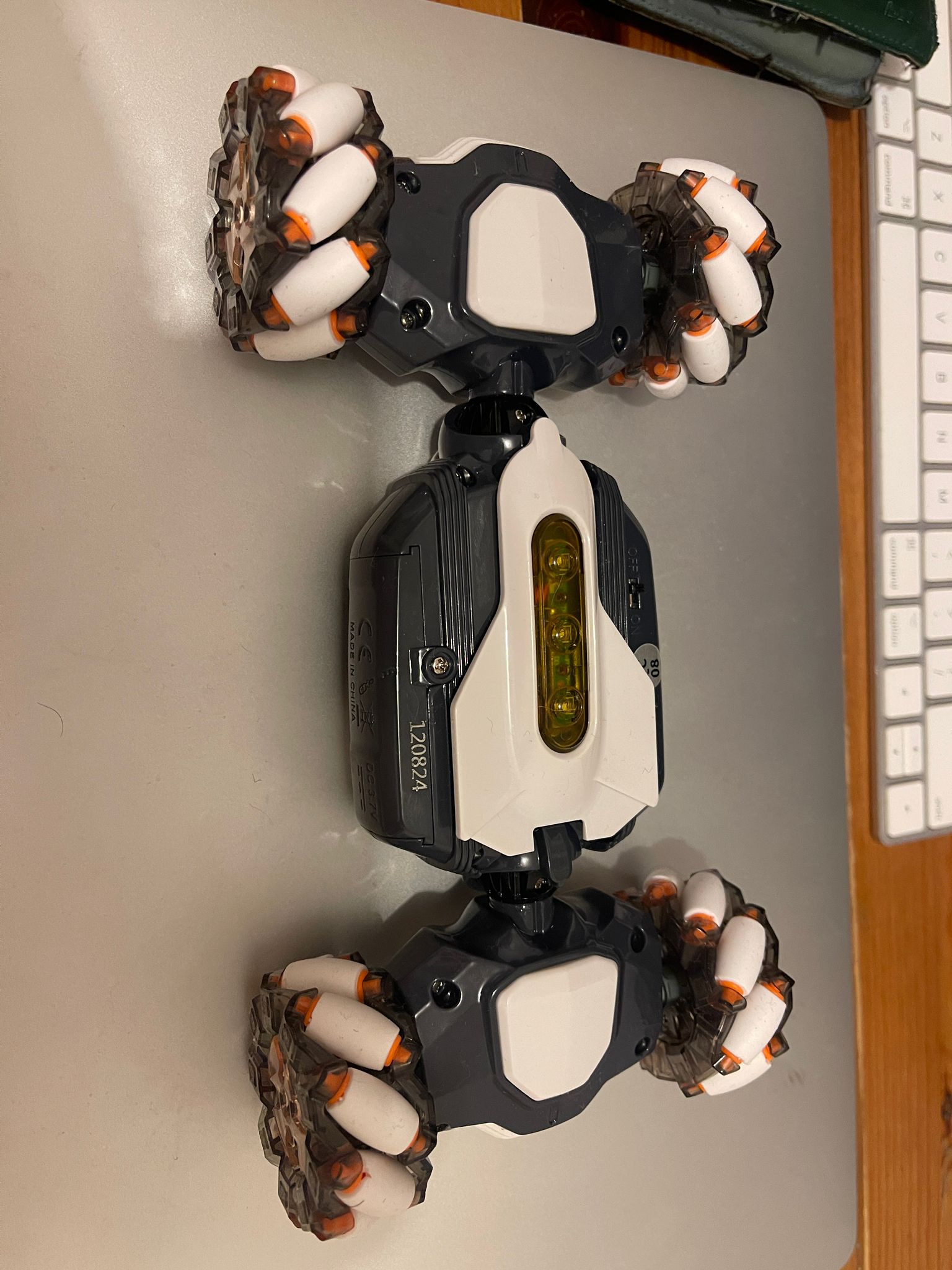
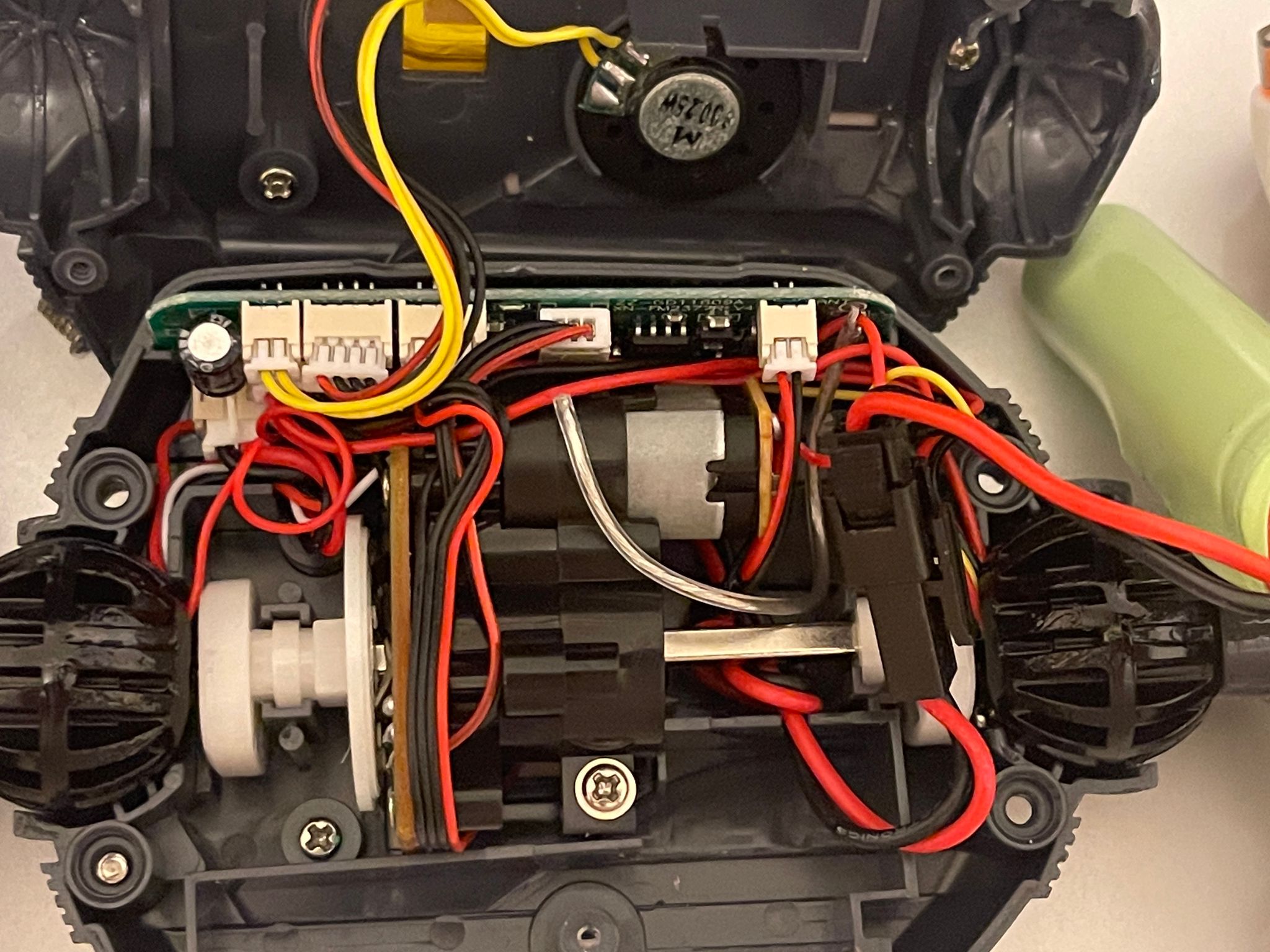
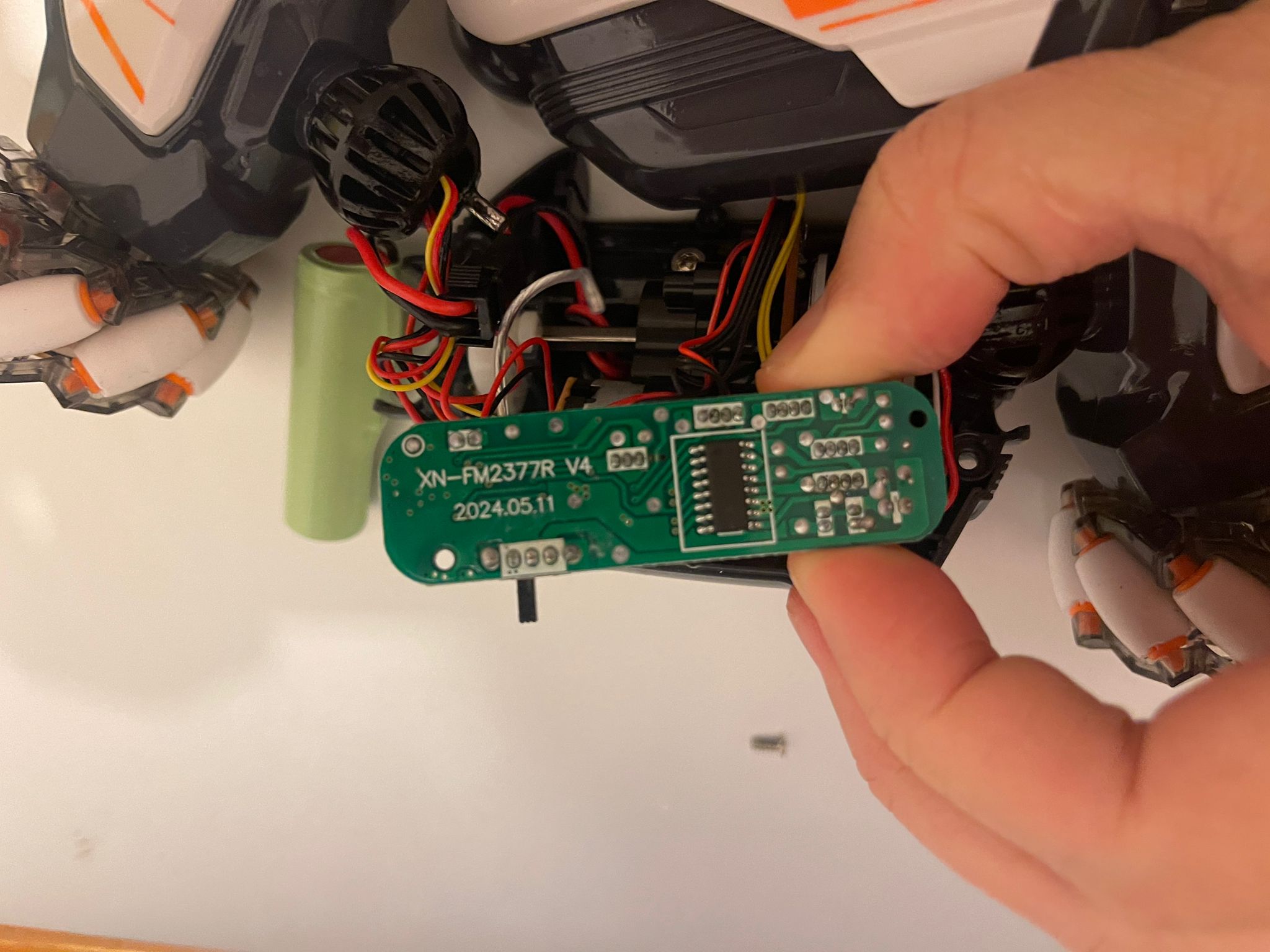
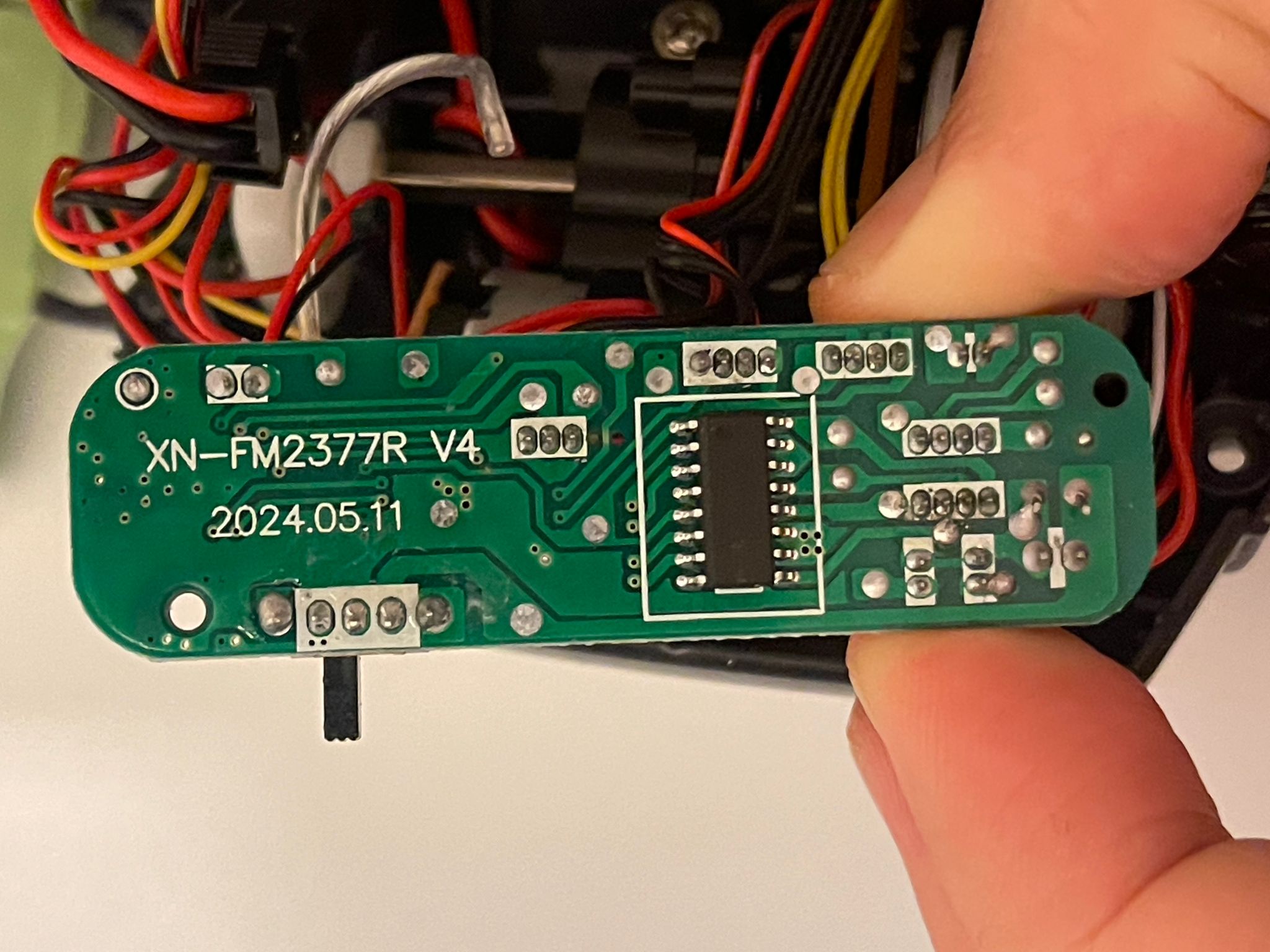
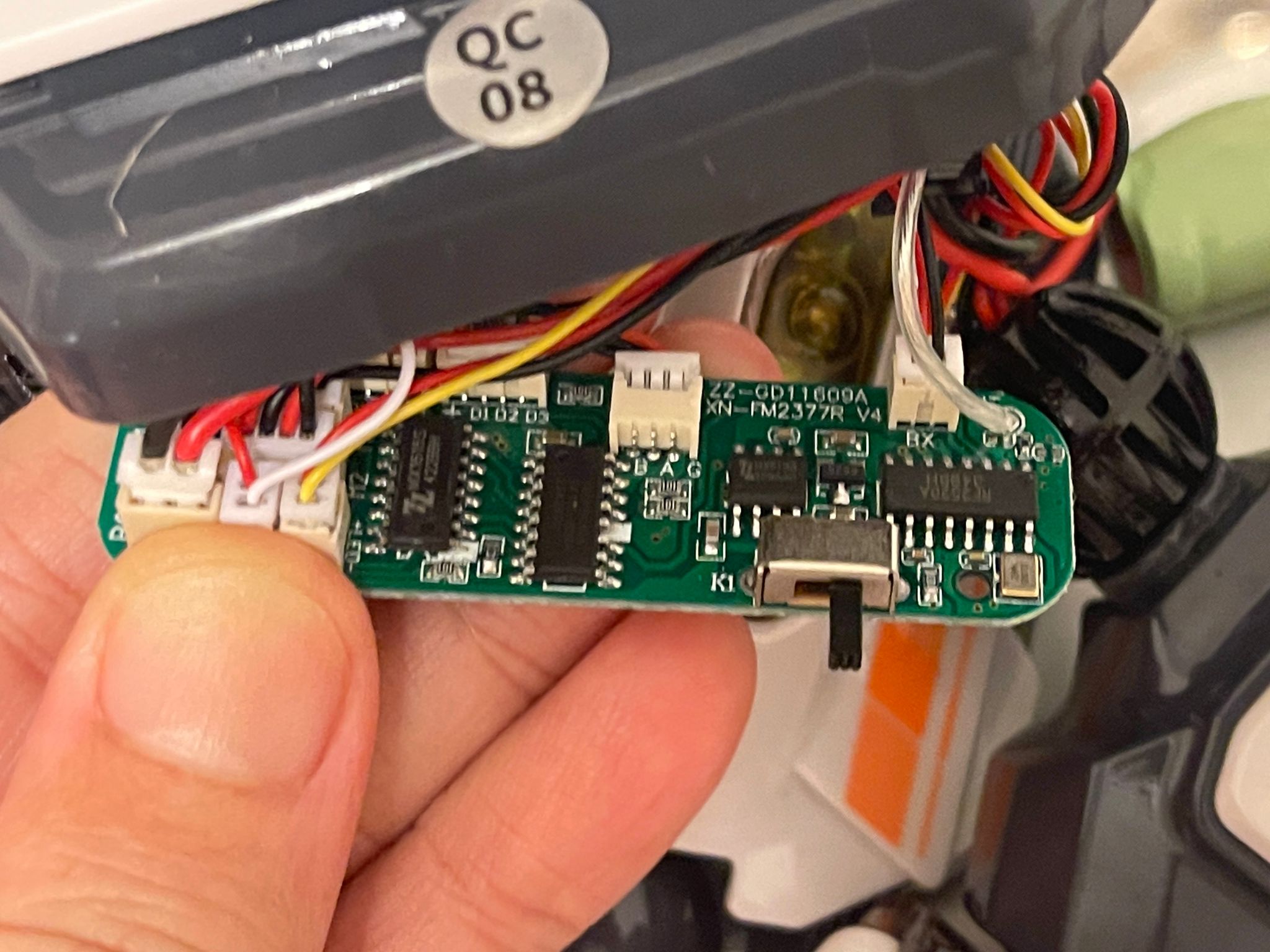
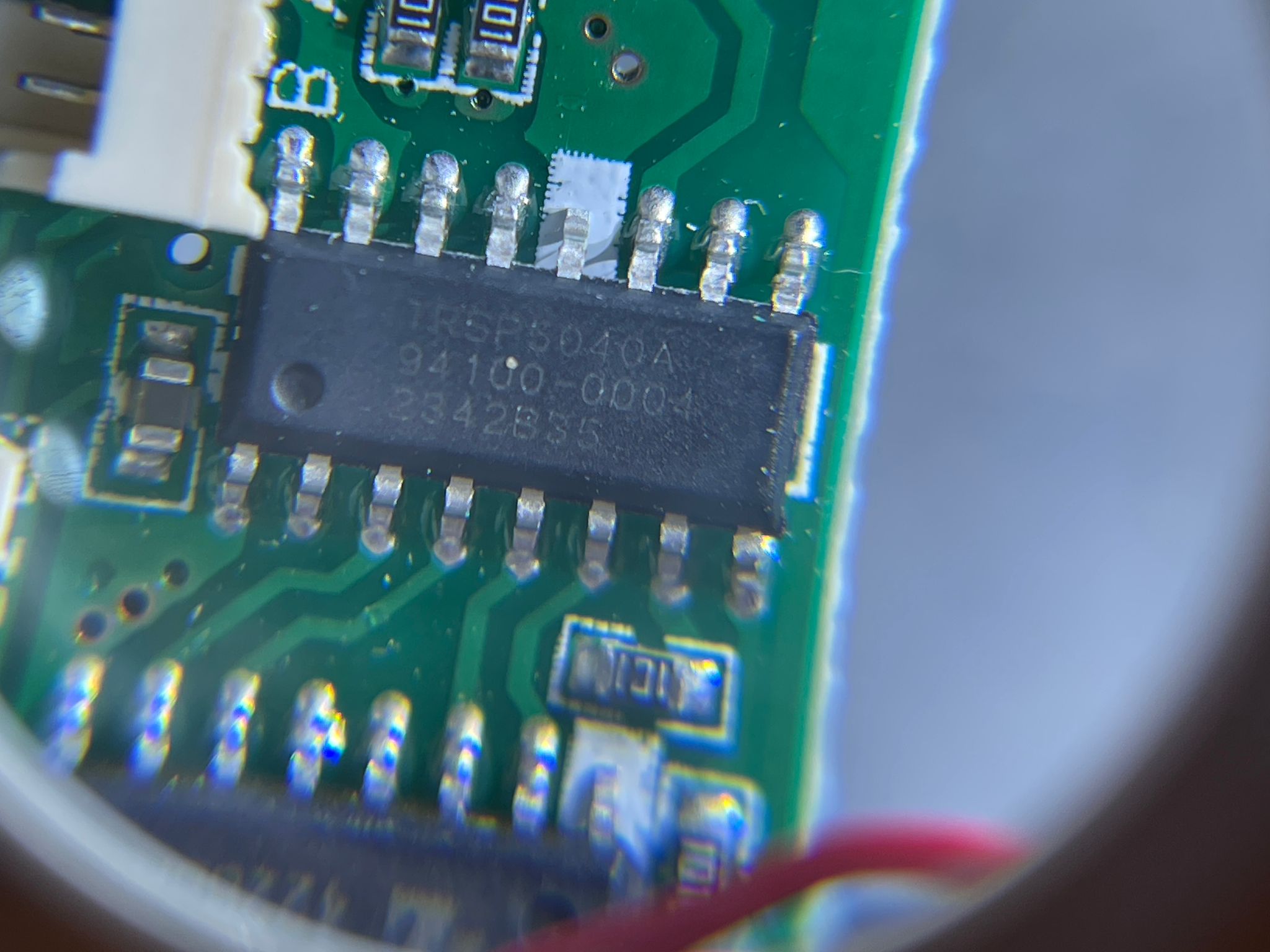
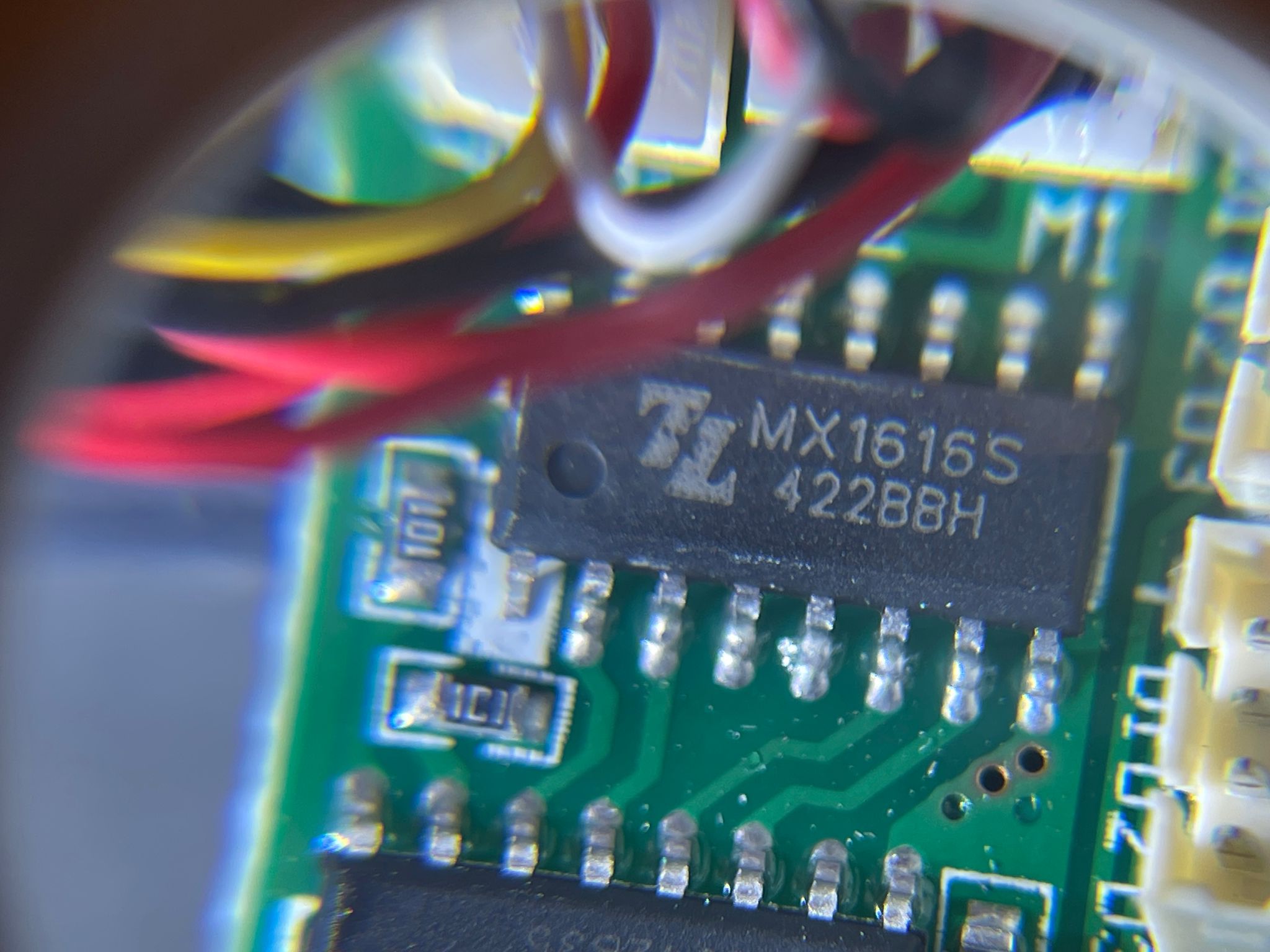
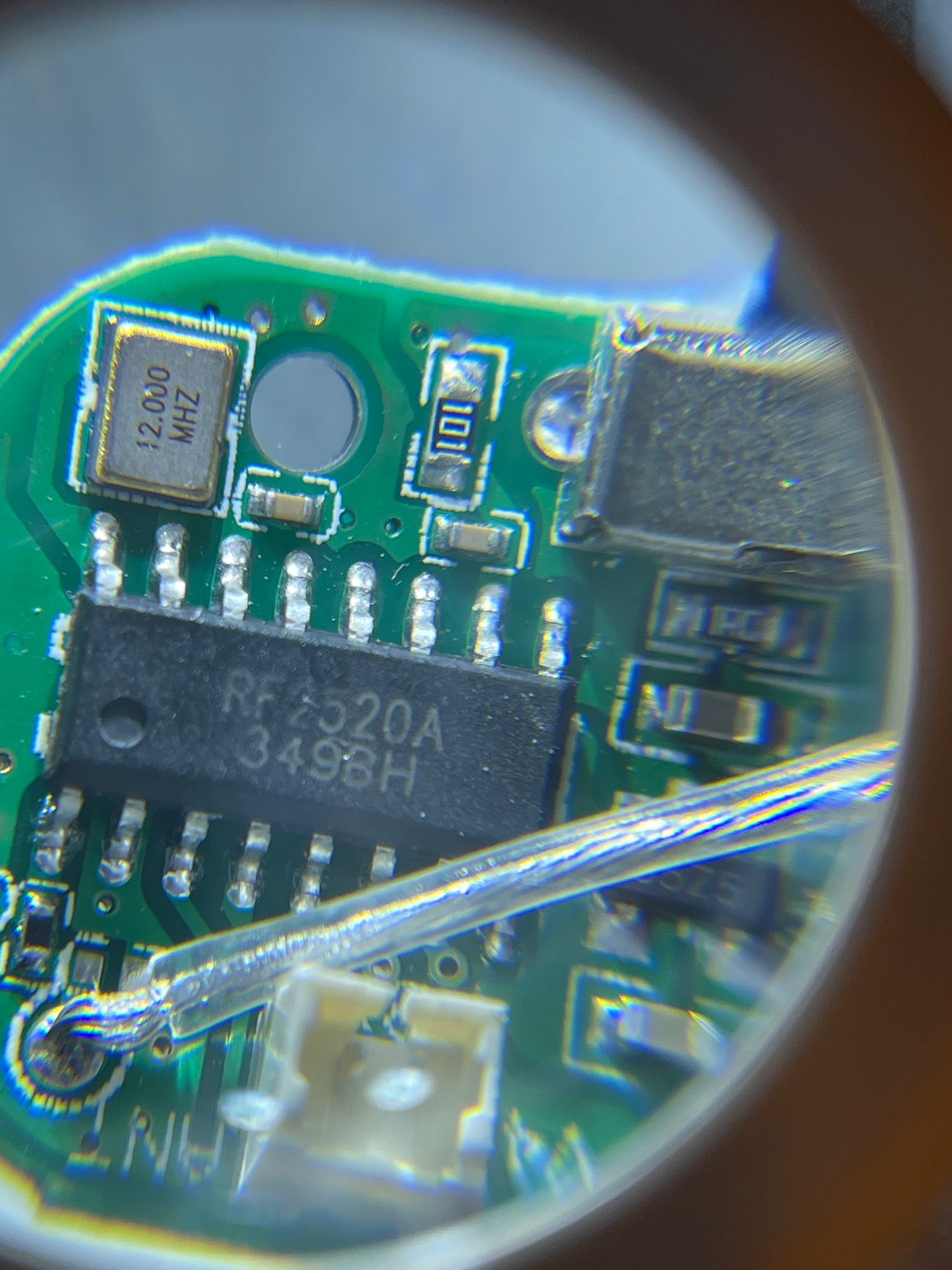
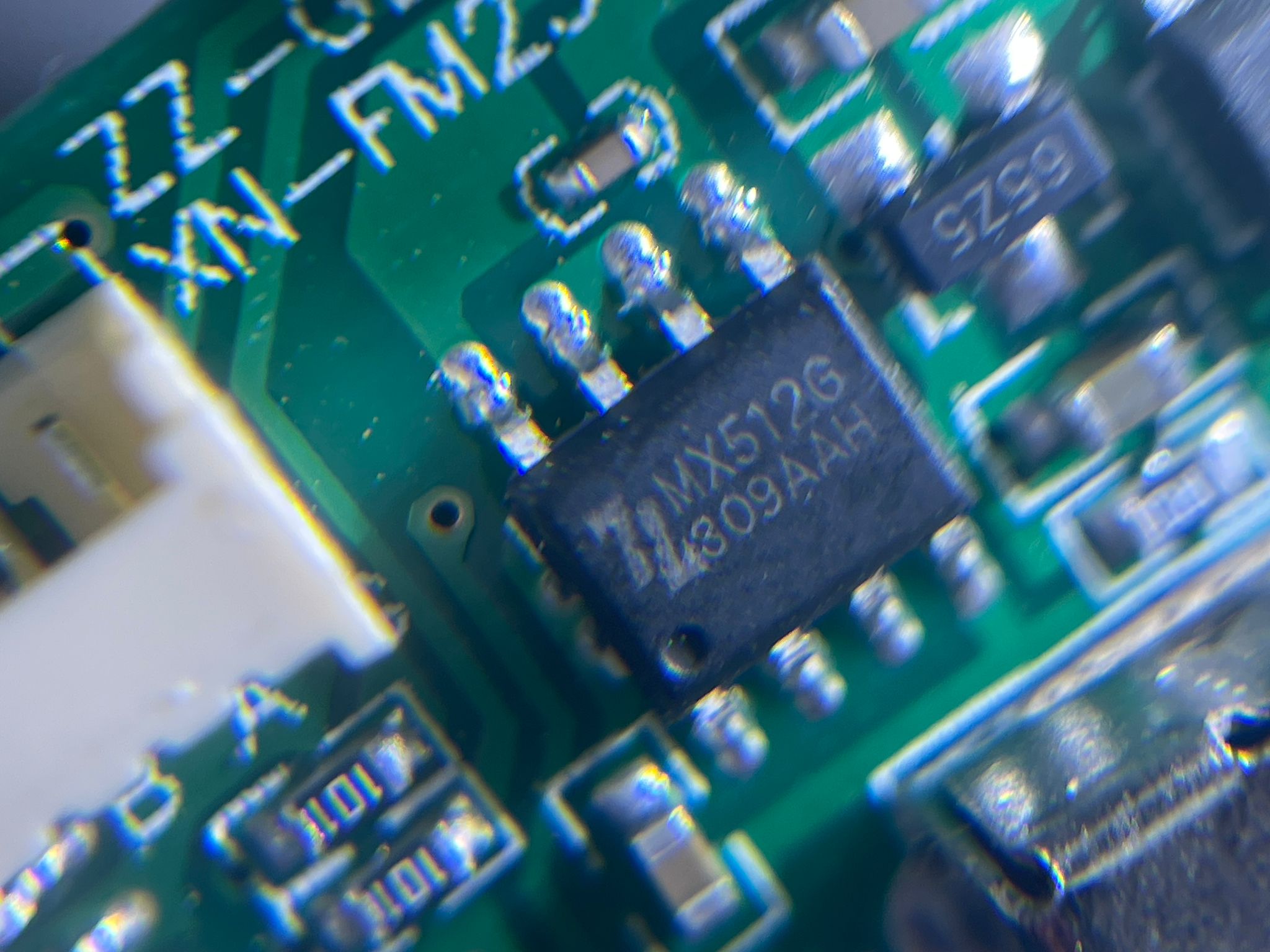
This tutorial give us a very good demo on rendering: https://www.youtube.com/watch?v=ukd1zO0Q-h4&t=317s
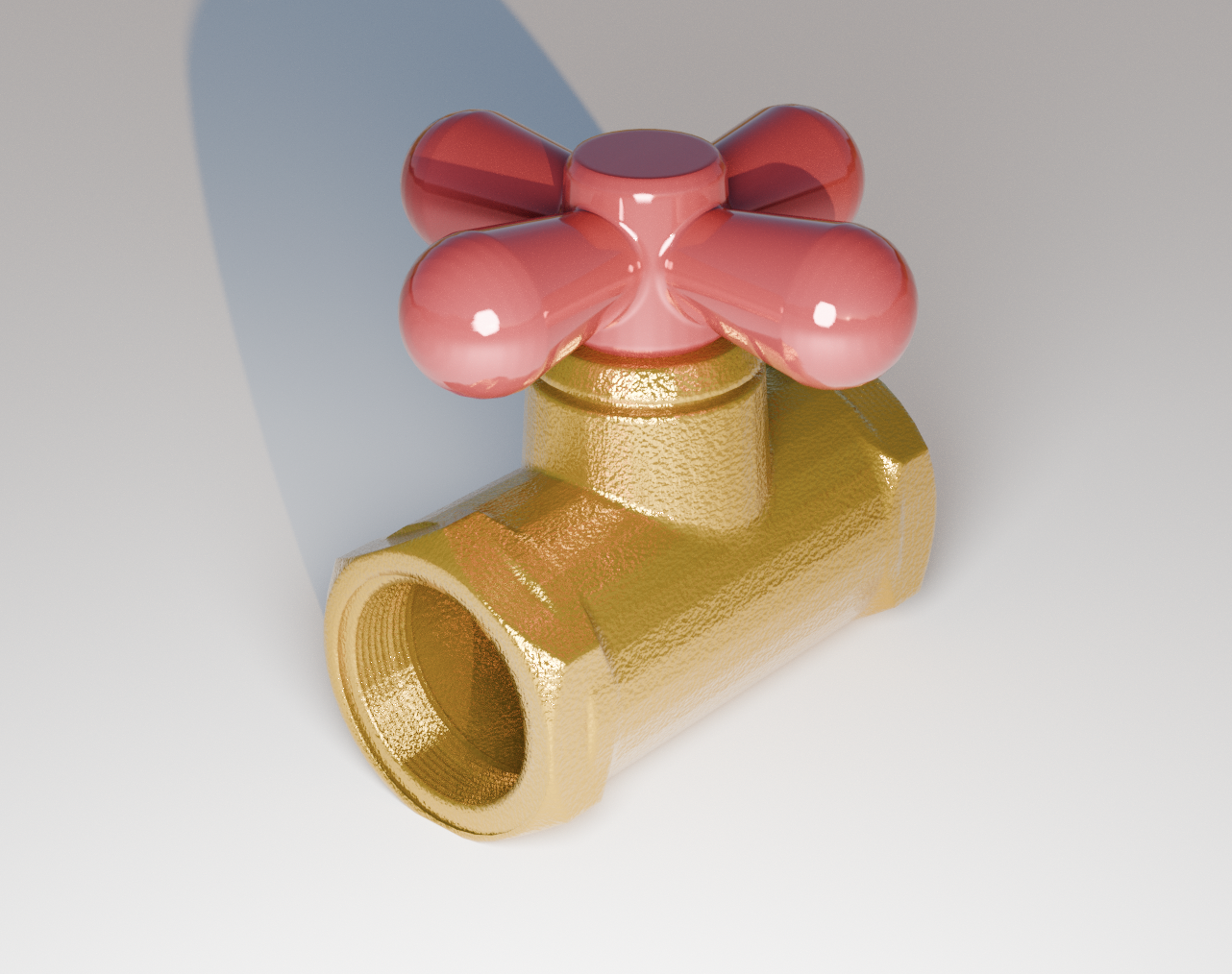
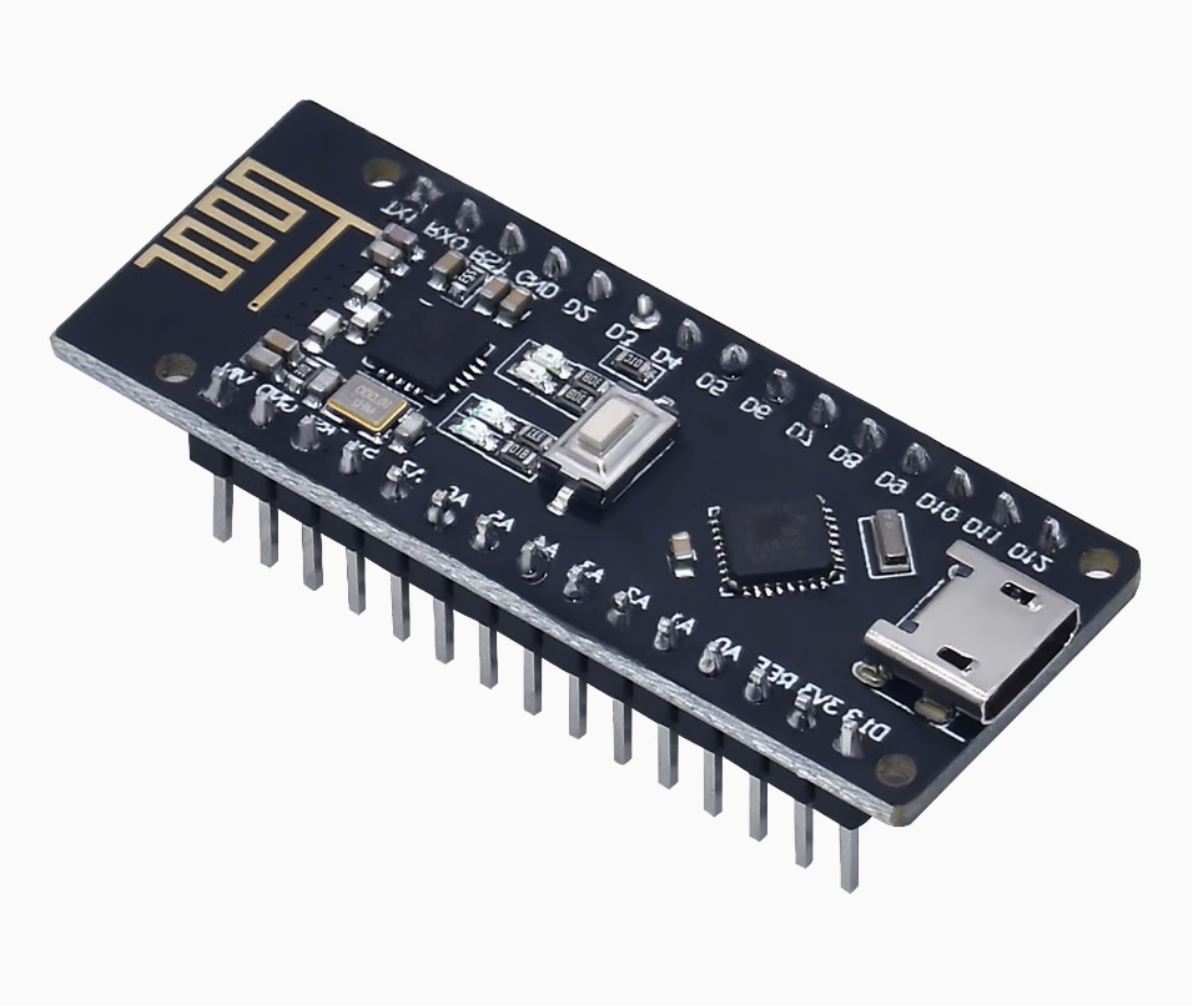
https://item.taobao.com/item.htm?_u=ibuhab0bb1b&id=628418040642&spm=a1z09.2.0.0.76e02e8dYbXP6k
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(100); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(100); // wait for a second
}
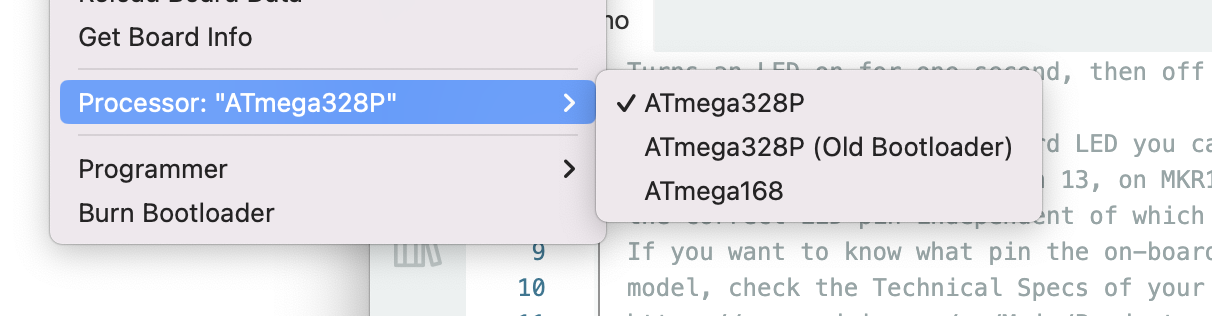
香港花太多時間係用玩具去吸引學生學編程,但無提升到對編程概念嘅表達,其實所有人都應該明白,如果係鐘意寫Program嘅小朋友,絕大部份第一次接觸就會愛上,其實係唔存在你要買好多玩具俾佢試先會所謂「啓發」到佢地嘅興趣。而個社會咁做不外乎得以下幾種可能
- 我係𡃁仔都不停叫阿媽買不同玩具玩啦,無接觸過編程嘅父母唔明白呢個道理所以不停買
- 因為賣玩具係一個賺錢嘅行業,而代理玩具黎香港賣亦都唔洗好有知識,自古以來香港係做左手交右手嘅生意,所以教育玩具業好發達。但真正對教育有研究嘅公司近乎無
- 老師要有舊見得到嘅野同校長交代,所以對接高編程技巧唔係首要,唔買舊玩具返黎又點有野睇呢,所以玩具業發達
上面三個原因互為影響互相加強,所以教育展變教育玩具展。
對香港嘅影響:
大家都係做代理唔研發,而大粒佬係呢啲展到都係得講野兩個字亦都唔會研發,最終後果就係香港洗好多錢係教育到最尾得個殼,香港人可以話俾外國人知香港投資左好多錢落教育,買左好多光鮮嘅玩具,除此之外乜都無。香港唔洗諗會出到Basic之父或者Logo之父等等一系列用實際行動去壓低學習難度嘅教育家,香港人唔洗諗,所以我地要以行動作出改變。
教育展中嘅清泉:
呢間公司做到好Deep,成套教學工具自己出,老細寫左本ROS嘅書有自己嘅學習思想,相信佢係用自己學習ROS嘅學習經驗濃縮出黎
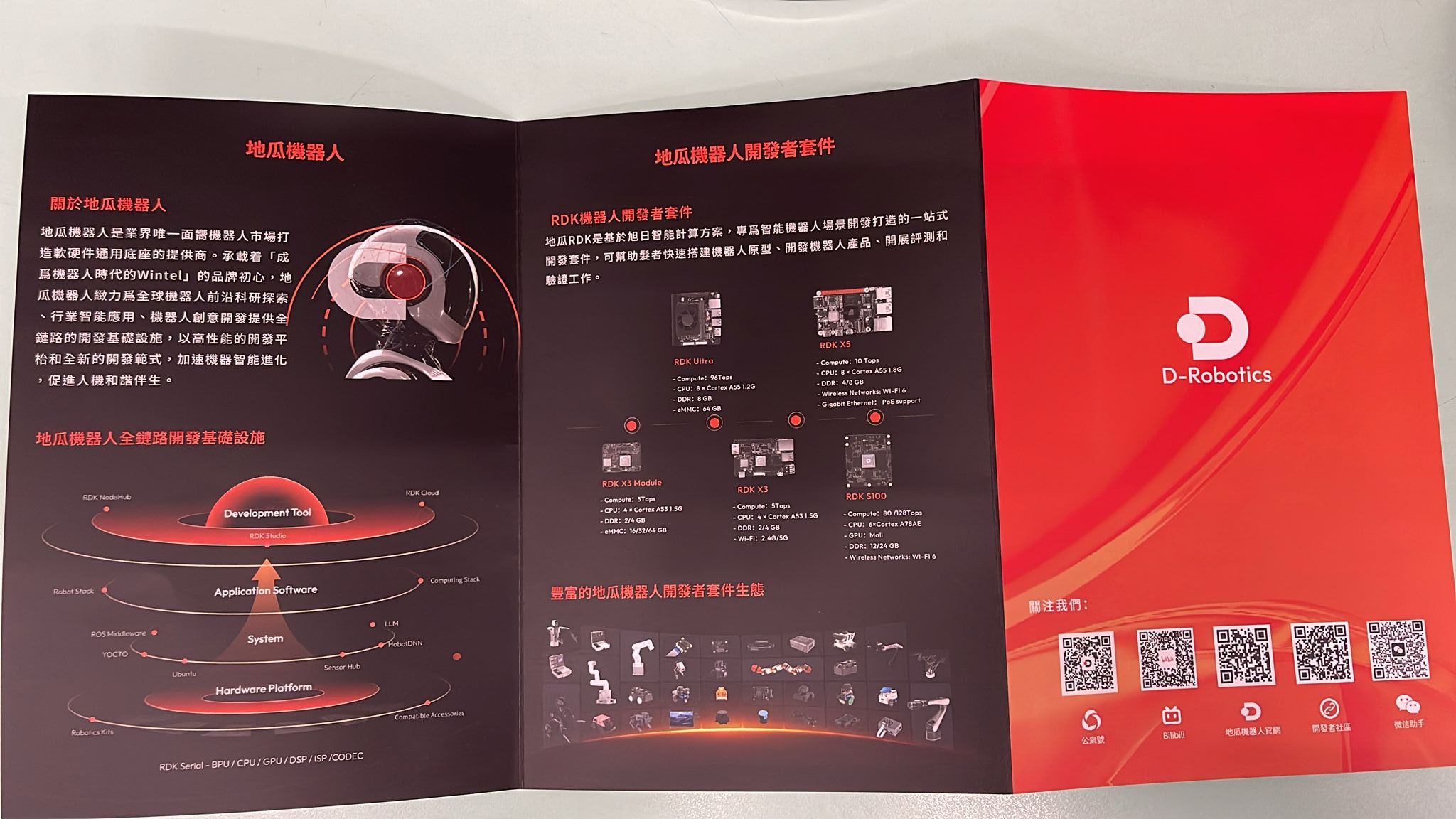
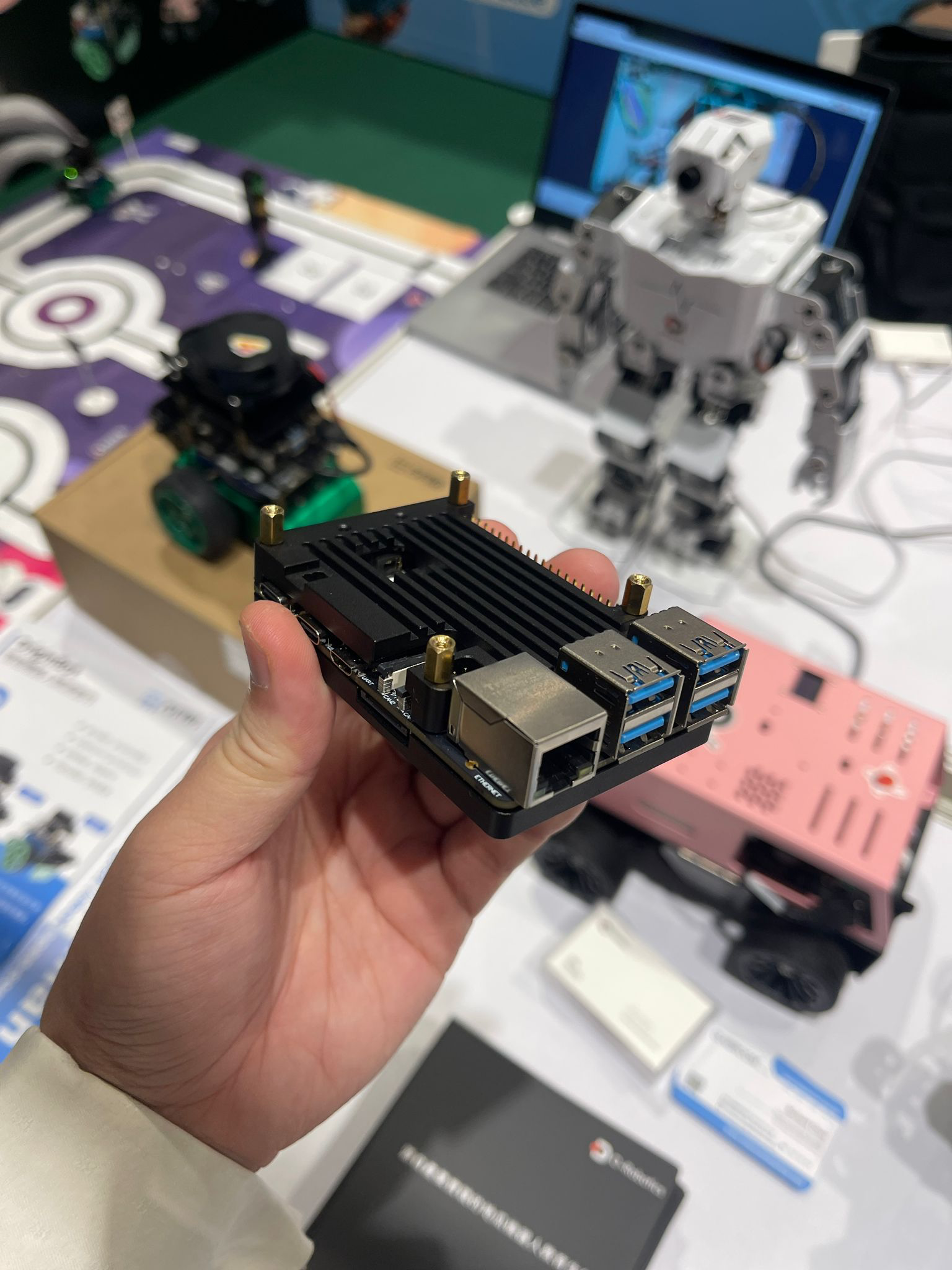
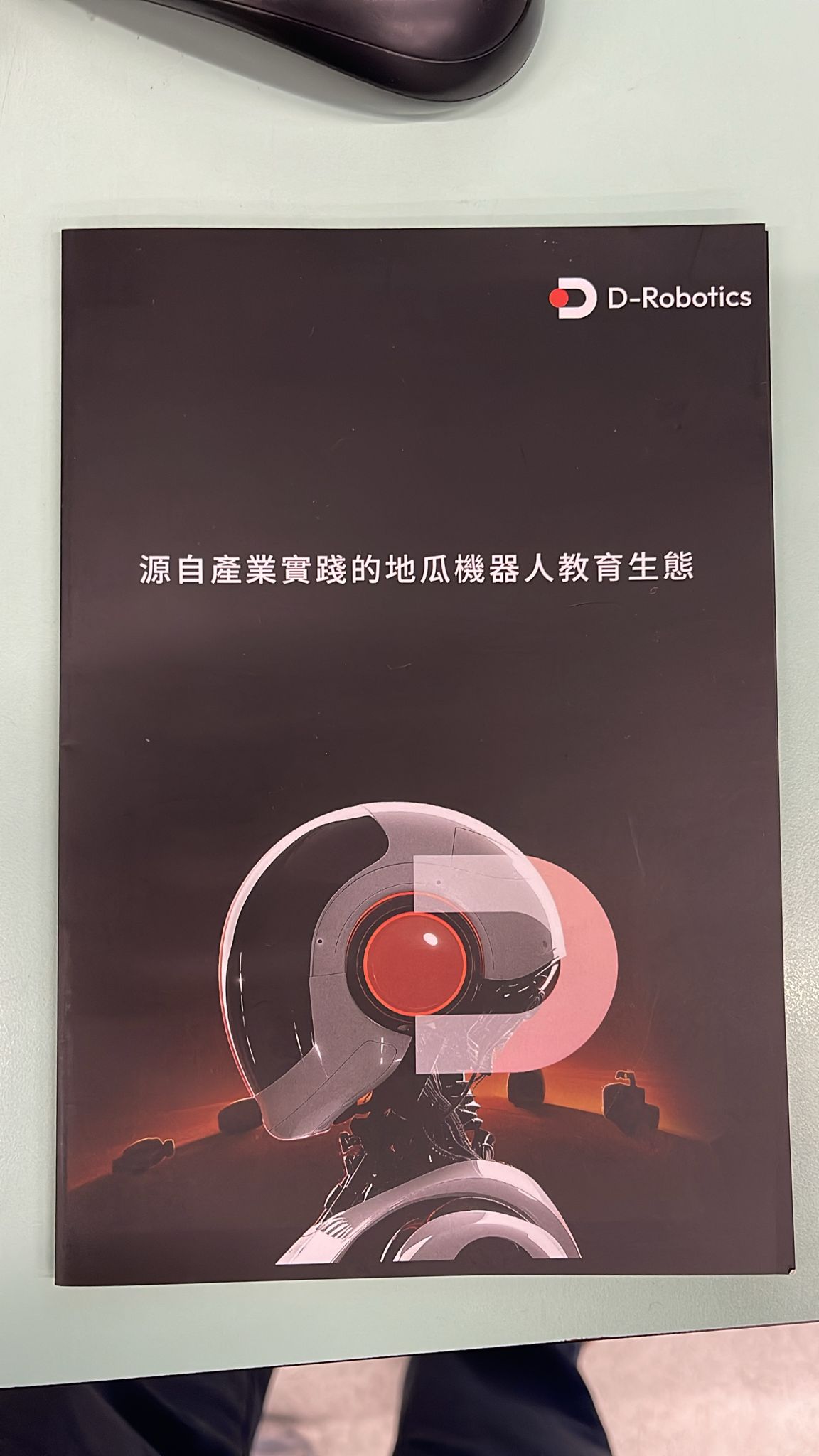
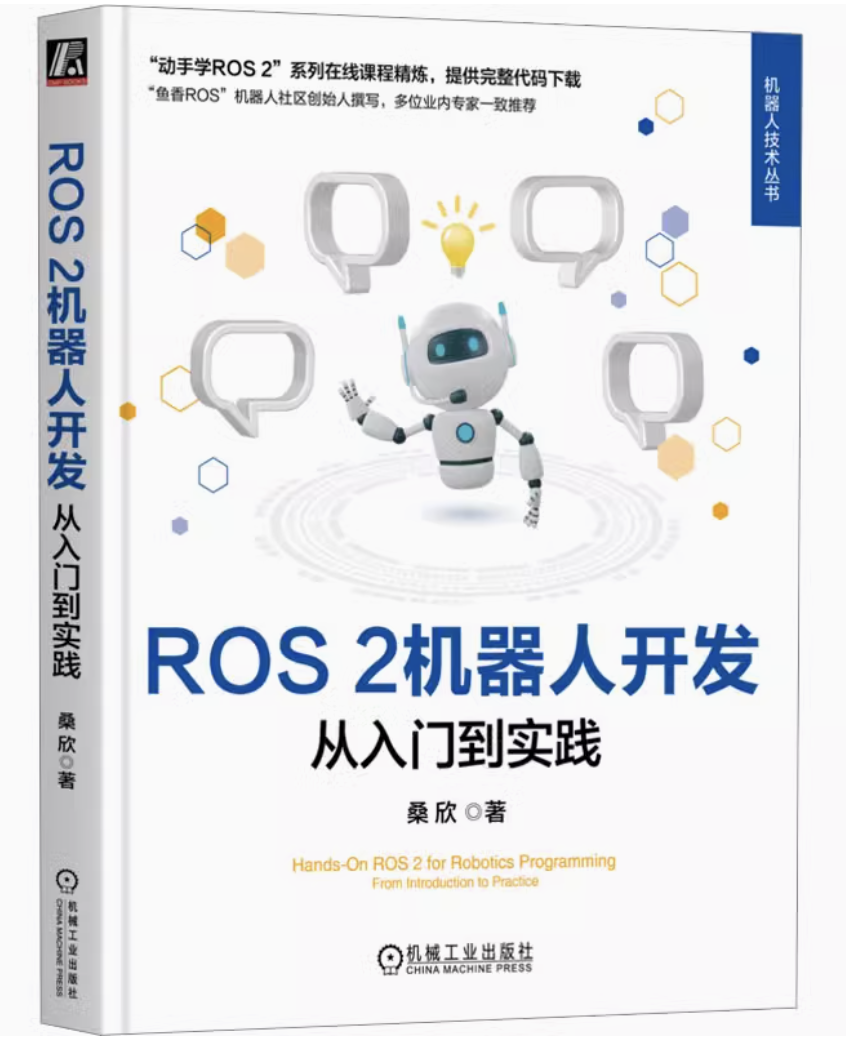
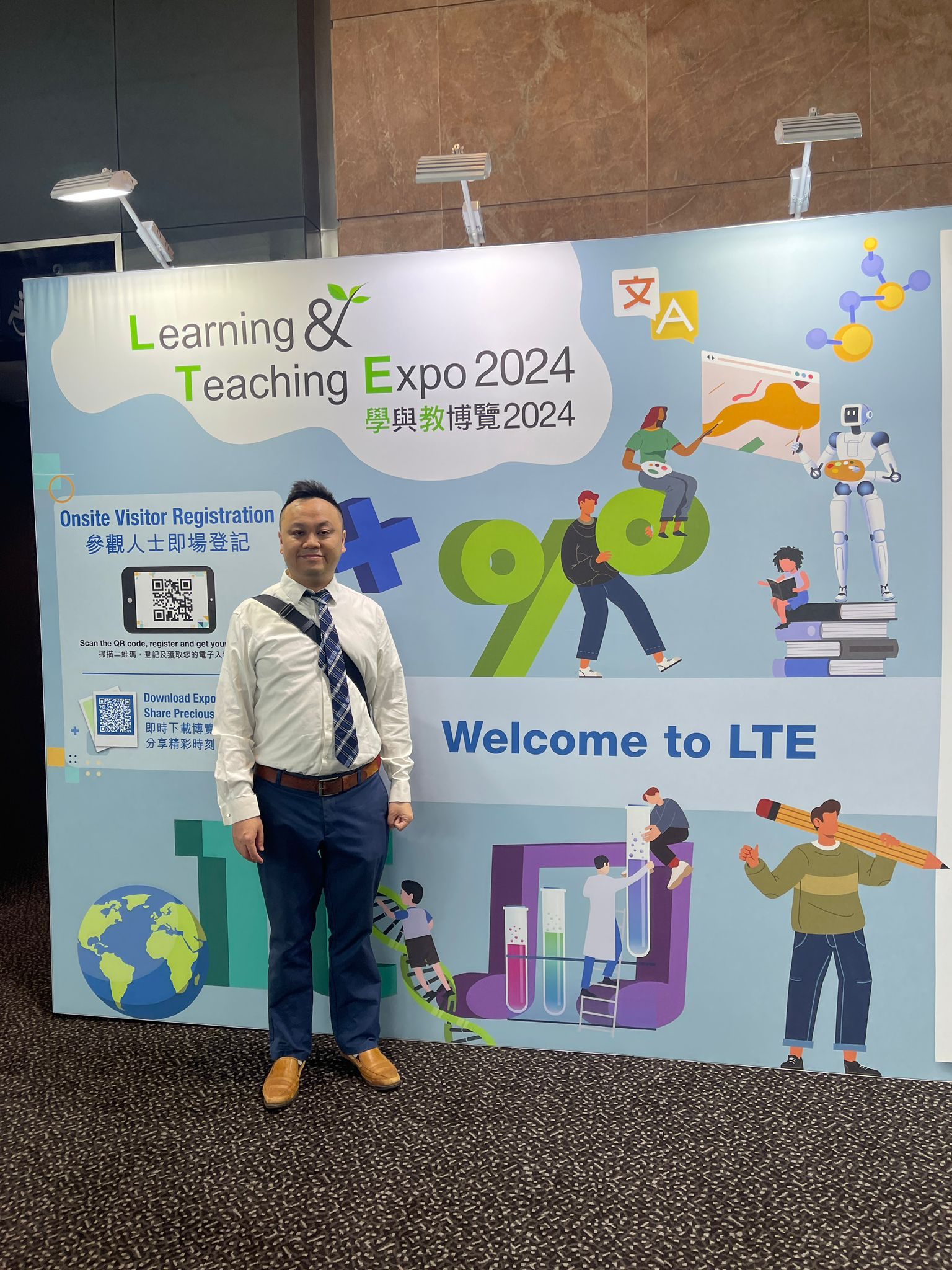
This function is using SharePoint-Java-API library.
public static HashSet<String> getAllFileOnSPO(Pair<String, String> token, String domain, String formDigestValue) throws UnsupportedEncodingException {
int pageSize = 5000;
int p_ID = 0;
HashSet<String> filenames = new HashSet();
int LastRow = 0;
String NextHref = null;
for (int z = 0; z < 1000; z++) {
String data = "{ \n"
+ " \"parameters\": {\n"
+ " \"RenderOptions\": 2,\n"
+ " \"ViewXml\": \"<View>"
+ "<Query>"
+ "<OrderBy>"
+ "<FieldRef Name=\\\"FileLeafRef\\\"/>"
+ "</OrderBy>"
+ "</Query>"
+ "<ViewFields>"
+ " <FieldRef Name='LinkFilename'/>"
+ "</ViewFields>"
+ "<RowLimit Paged=\\\"TRUE\\\">" + pageSize + "</RowLimit>"
+ "</View>\"\n"
+ " "
+ " }"
+ "}";
// System.out.println(data);
JSONObject json2 = new JSONObject(data);
String url;
if (NextHref == null) {
url = "/sites/DMS/_api/web/GetListUsingPath(DecodedUrl=%27%2Fsites%2FDMS%2FAccount%27)/RenderListDataAsStream?RootFolder=" + URLEncoder.encode("/sites/DMS/", "utf-8") + "&PageFirstRow=" + (z * pageSize) + "&p_ID=" + p_ID + "&Paged=TRUE&ix_Paged=TRUE&ix_ID=" + p_ID;
} else {
url = "/sites/DMS/_api/web/GetListUsingPath(DecodedUrl=%27%2Fsites%2FDMS%27)/RenderListDataAsStream" + NextHref;
}
System.out.println("url=" + url);
String jsonString = SPOnline.post(token, domain, url, data, formDigestValue);
json2 = new JSONObject(jsonString);
System.out.println("length=" + json2.getJSONArray("Row").length());
System.out.println(" RowLimit=" + json2.getInt("RowLimit"));
System.out.println(" LastRow=" + json2.getInt("LastRow"));
LastRow = json2.getInt("LastRow");
JSONArray arr = json2.getJSONArray("Row");
if (arr.length() == 0) {
break;
}
for (int x = 0; x < arr.length(); x++) {
JSONObject obj = arr.getJSONObject(x);
filenames.add(obj.getString("FileLeafRef"));
}
p_ID = arr.getJSONObject(arr.length() - 1).getInt("ID");
if (!json2.has("NextHref")) {
break;
}
System.out.println(" NextHref=" + json2.getString("NextHref"));
NextHref = json2.getString("NextHref");
}
return filenames;
}
If your c# azure functions project in visual studio has error : “There is no Functions runtime available that matches the version specified in the project.”. Do this :
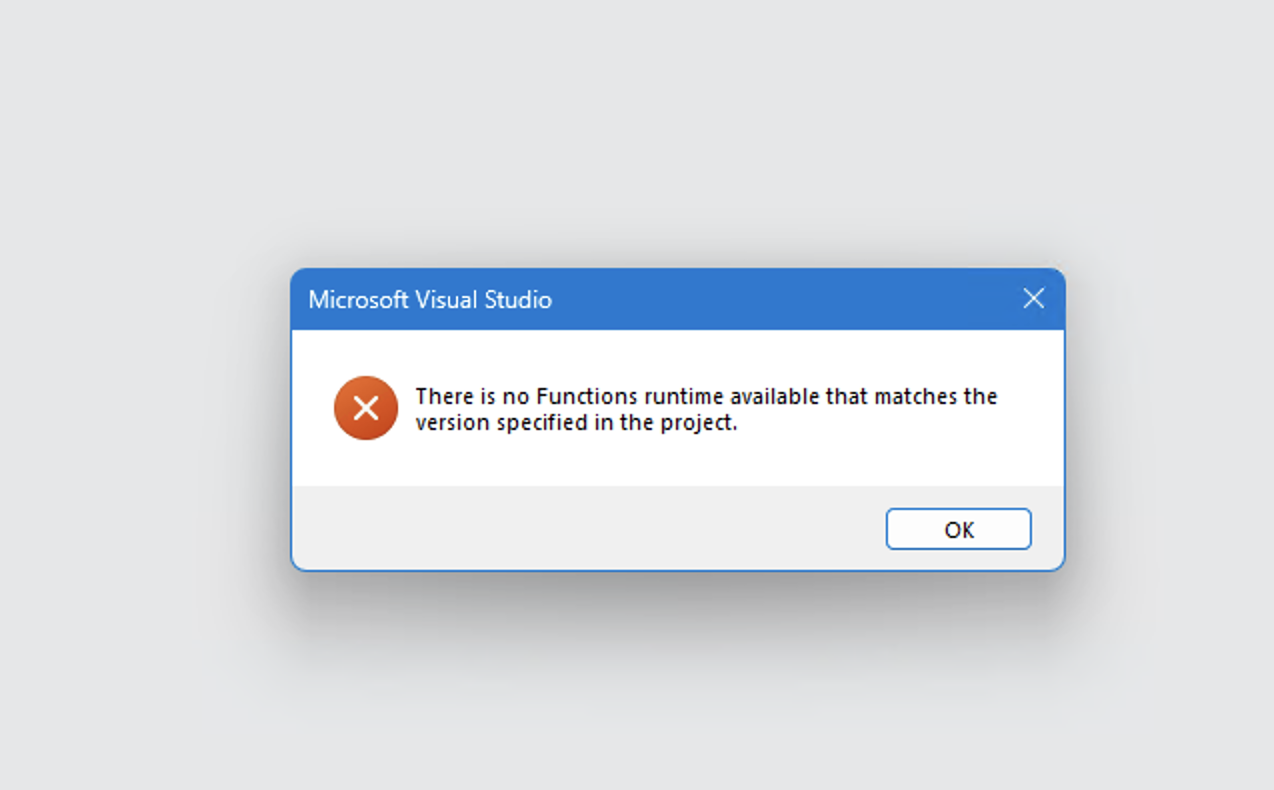
Click : Download & Install
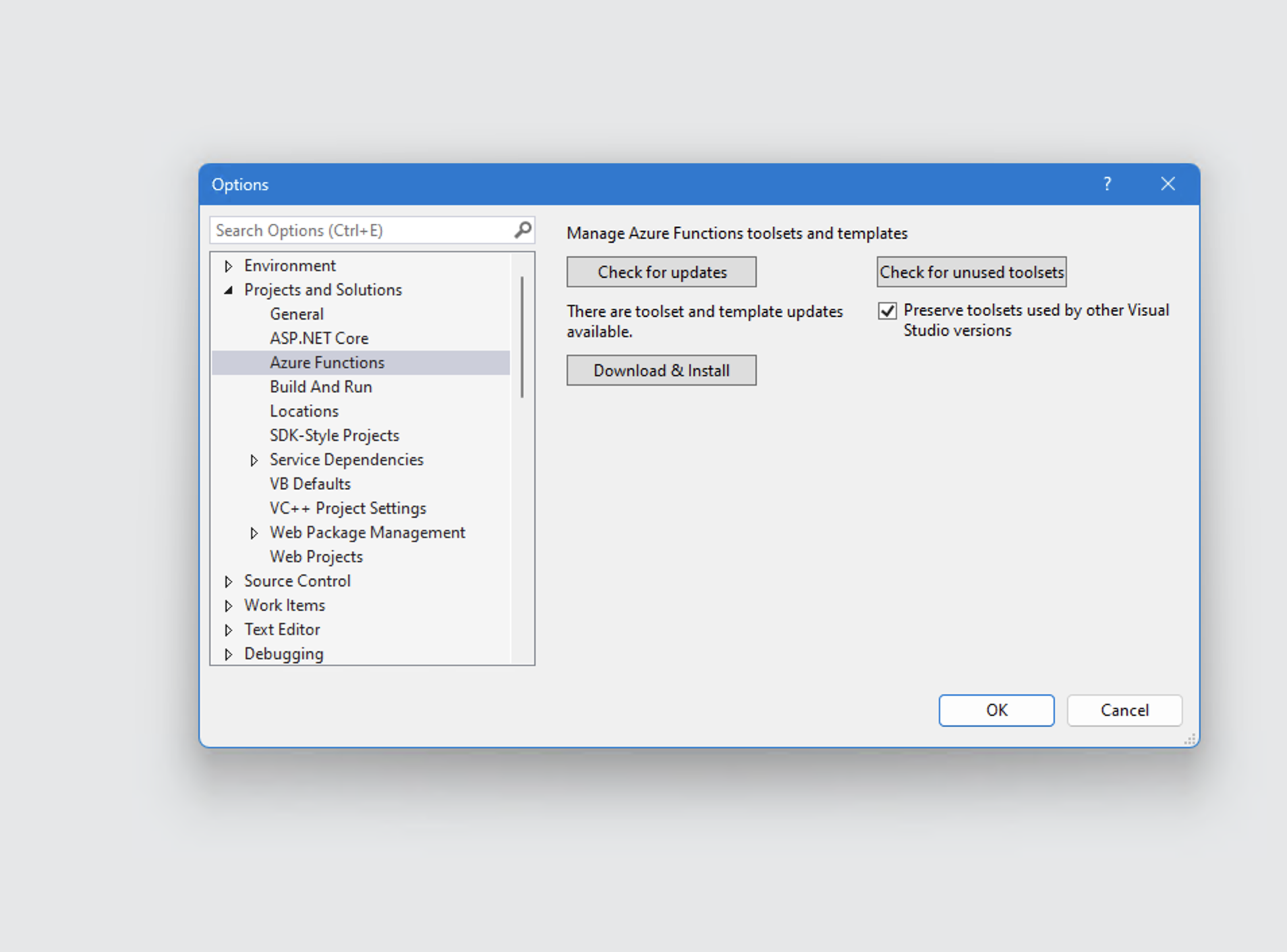
Run this command in windows host
netsh interface portproxy add v4tov4 listenport=22 listenaddress=0.0.0.0 connectport=22 connectaddress=<wsl ubuntu ip>
To enable debug, “CFLAGS=-g ./configure” won’t work, edit ./isa/Makefile and add -g to variable RISCV_GCC_OPTS then it works.
The dump file won’t show debug info, run “riscv64-unknown-elf-objdump -DS rv64ui-v-addi” then you see it
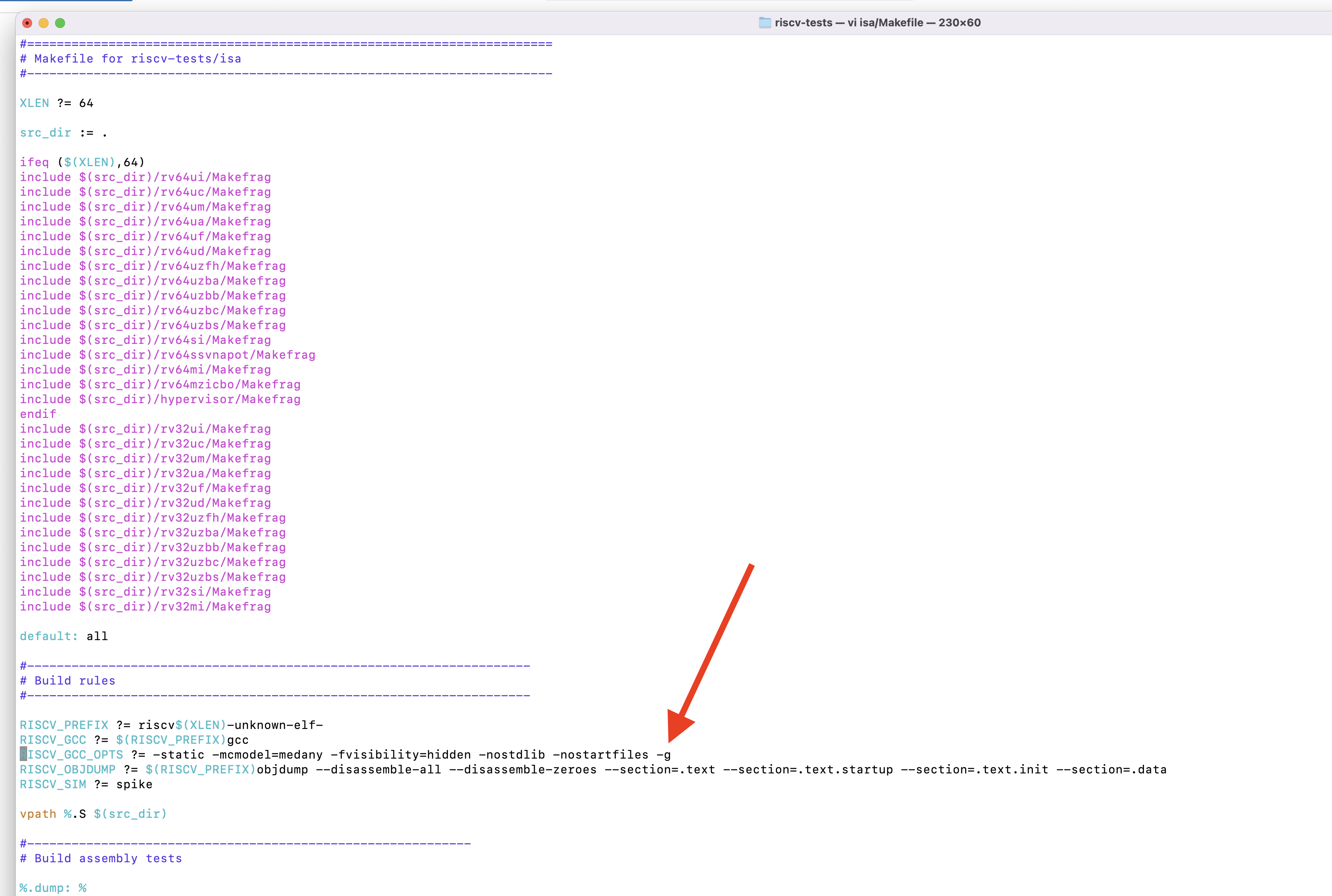
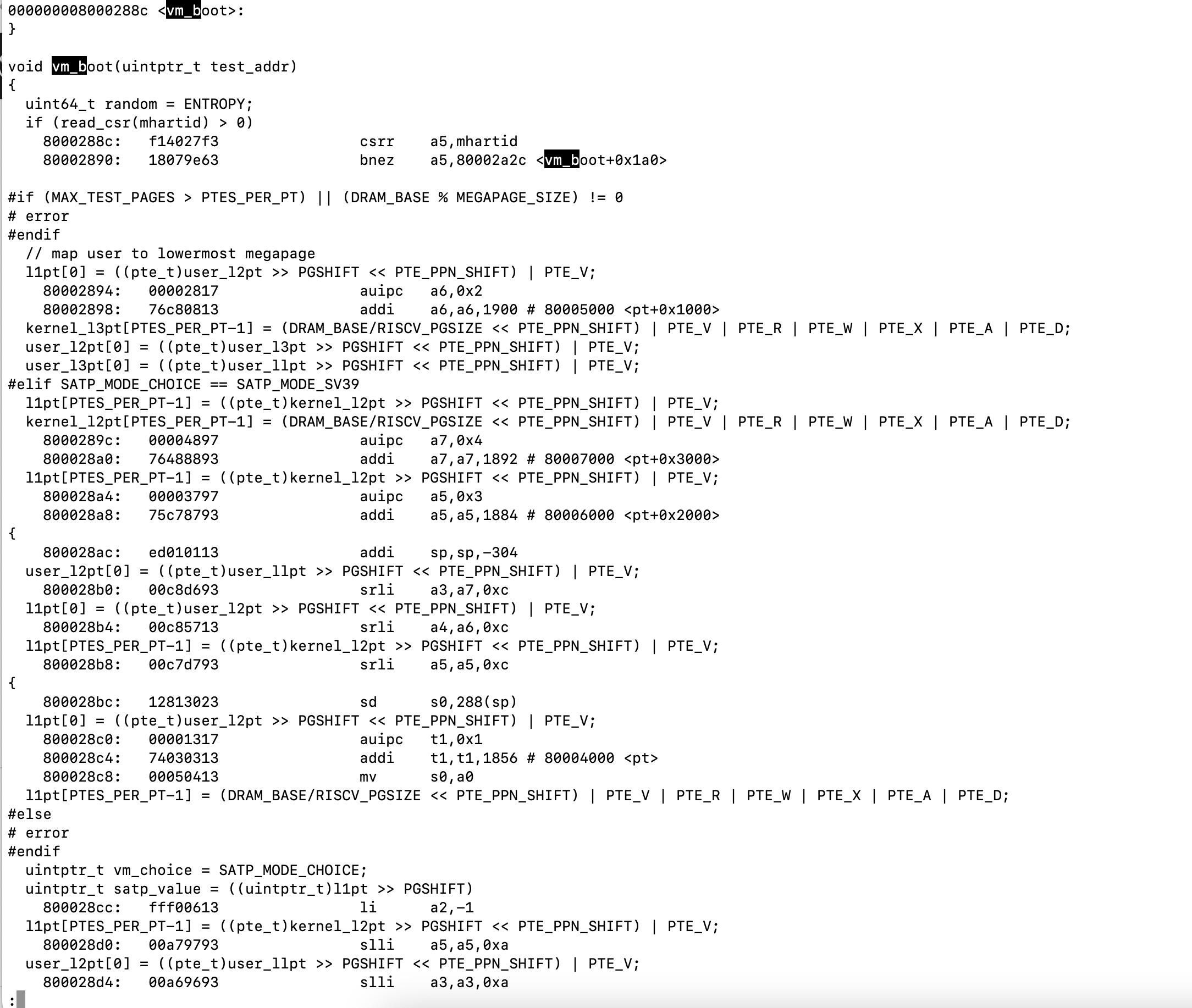
The technique they have used are “trait“, “magic function” and “call_user_func_array“. The whole thing work in runtime, not compile time.
1. Php trait
PHP trait is just like copy and paste the code from trait into the injected class.
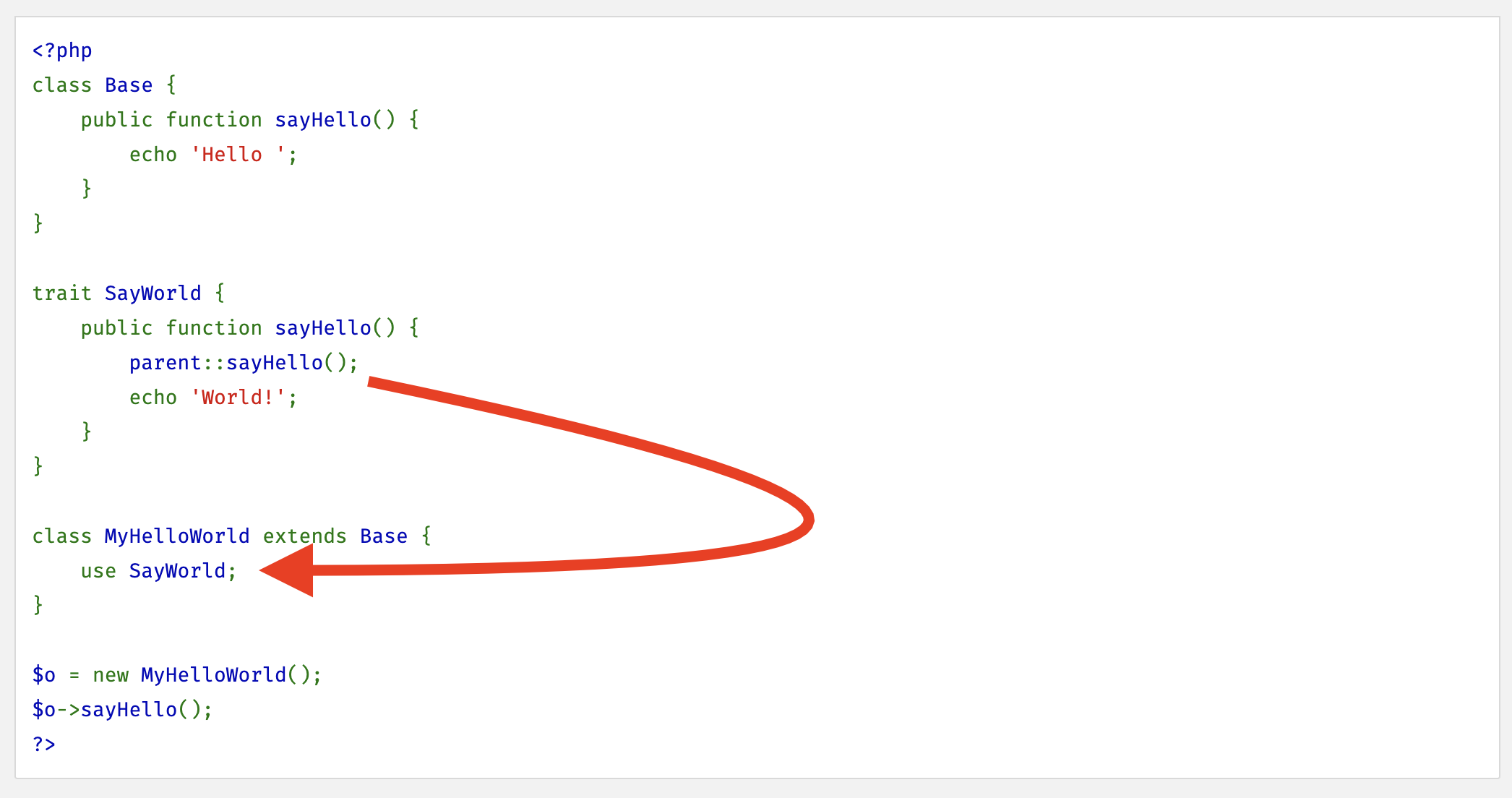
2. Magic function
When calling a not exist function, the __call function will be called. Laravel is using library Macroable, so in the code, when you are calling a non exist function on an object, the __call will be called and try to call the real function by call_user_func_array
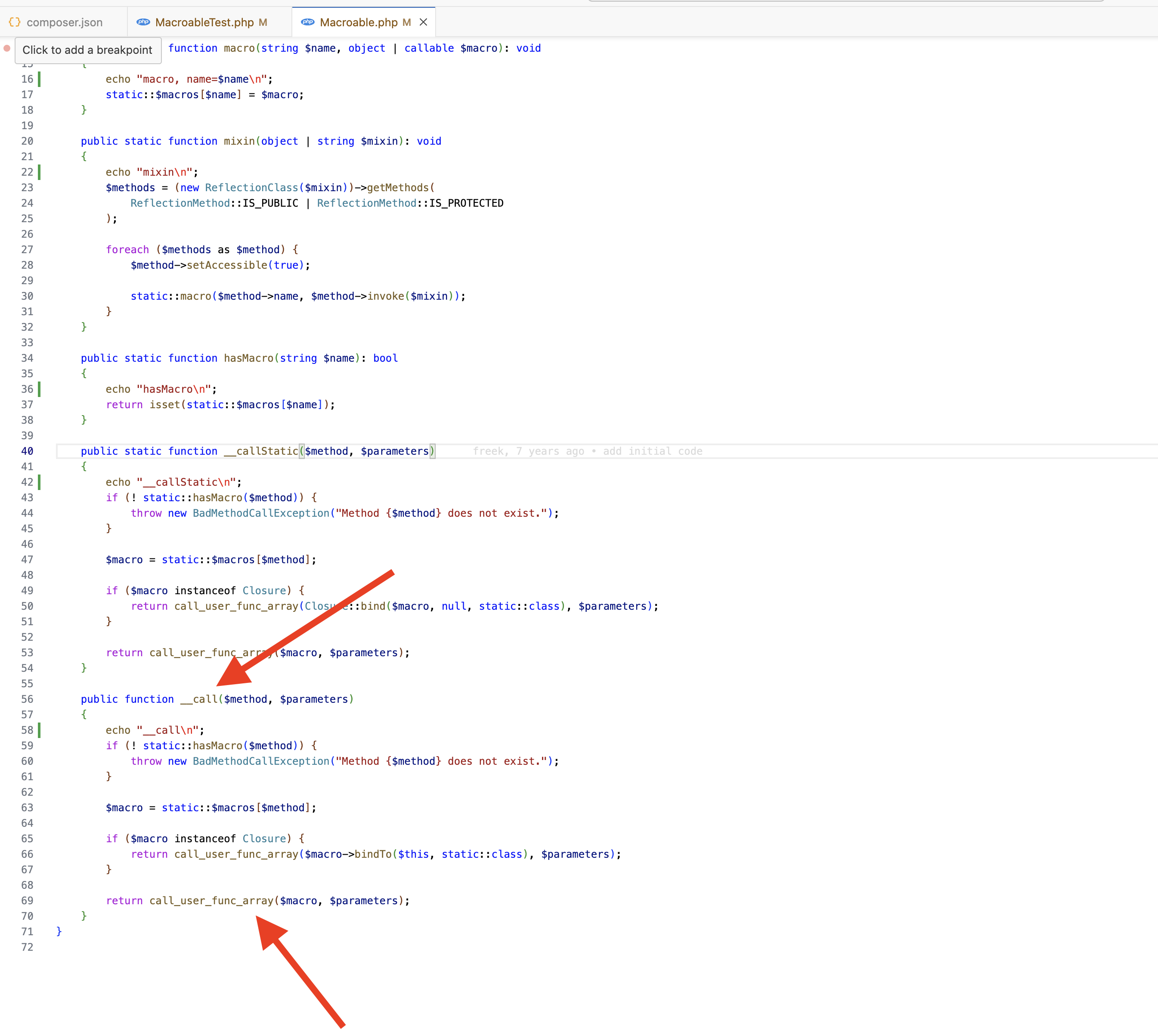
this problem happens in both mac and ubuntu
/home/peter/workspace/riscv-tests/benchmarks/dhrystone/dhrystone.c:20:1: error: return type defaults to ‘int’ [-Wimplicit-int]
Fixed is here https://github.com/riscv-software-src/riscv-tests/compare/master…pratikkedar:riscv-tests:pratik-test
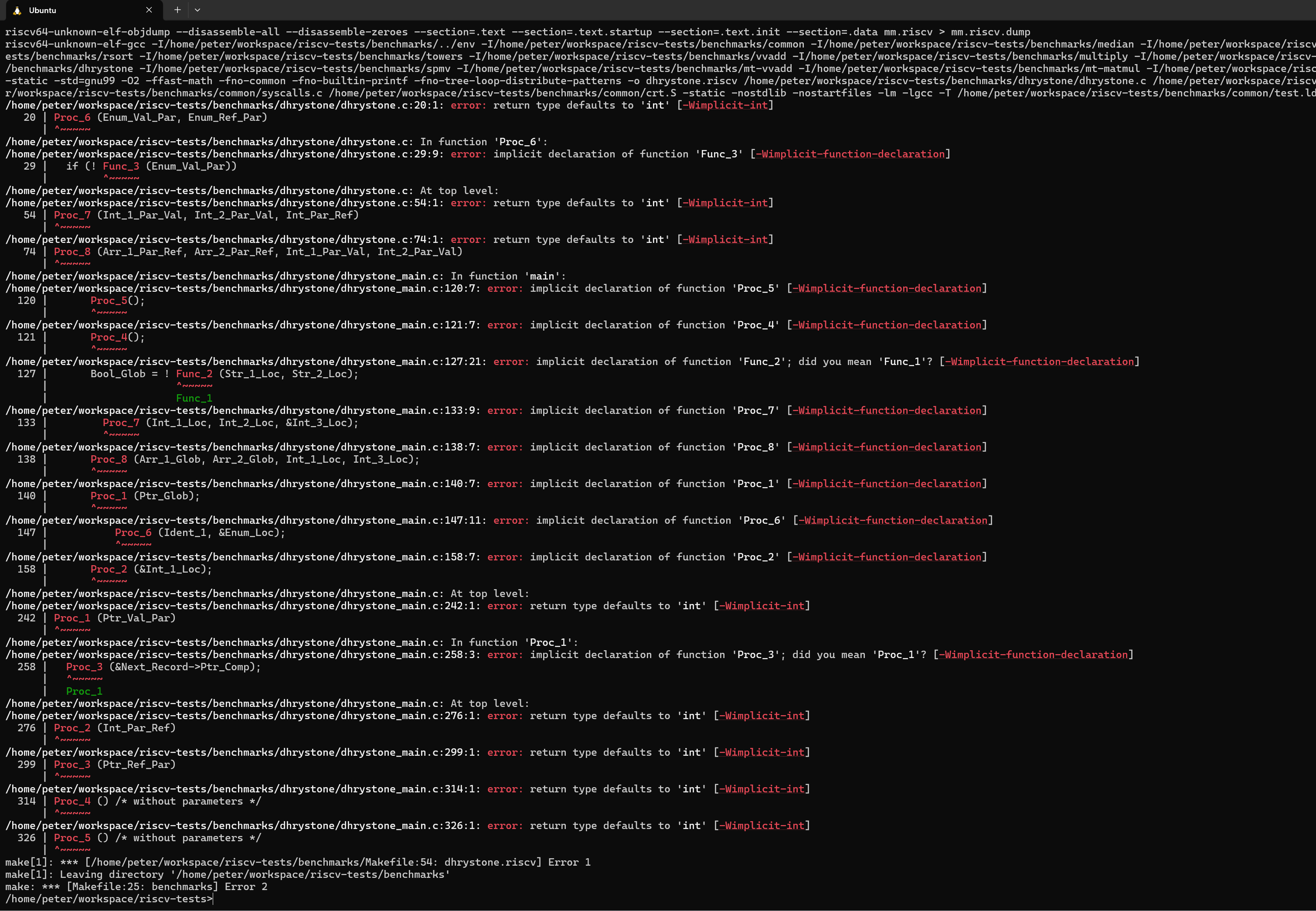
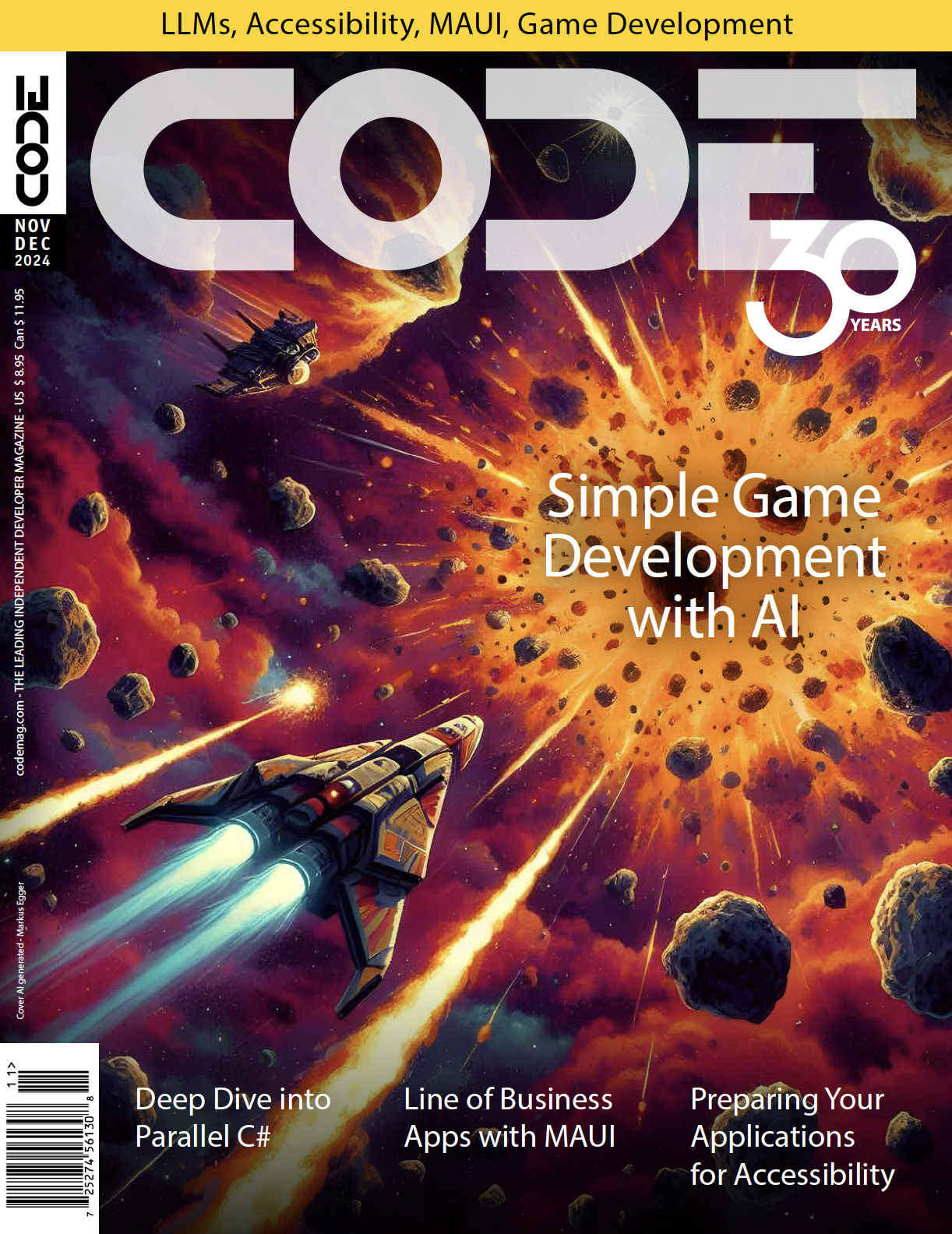
Total page | Total article | Editorial (no of page) | Longest article (no of page) | Advertisement (no of page) | |
2024 Nov Dec | 76 | 11 | 1 | 14 | 5 |
2024 Sep Oct | 76 | 12 | 1 | 25 | 8 |
if you meet this problem: fatal: the remote end hung up unexpectedly
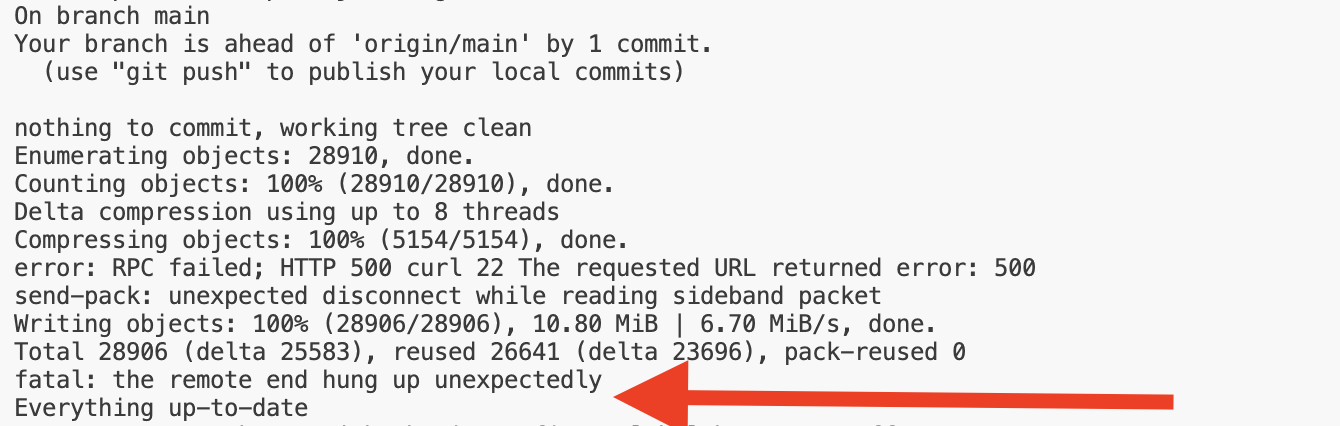
Do this:
git config --global http.postBuffer 157286400
This book is totally outdated because the official facebook PHP SDK is already deprecated. Now we should go for facebook javascript SDK
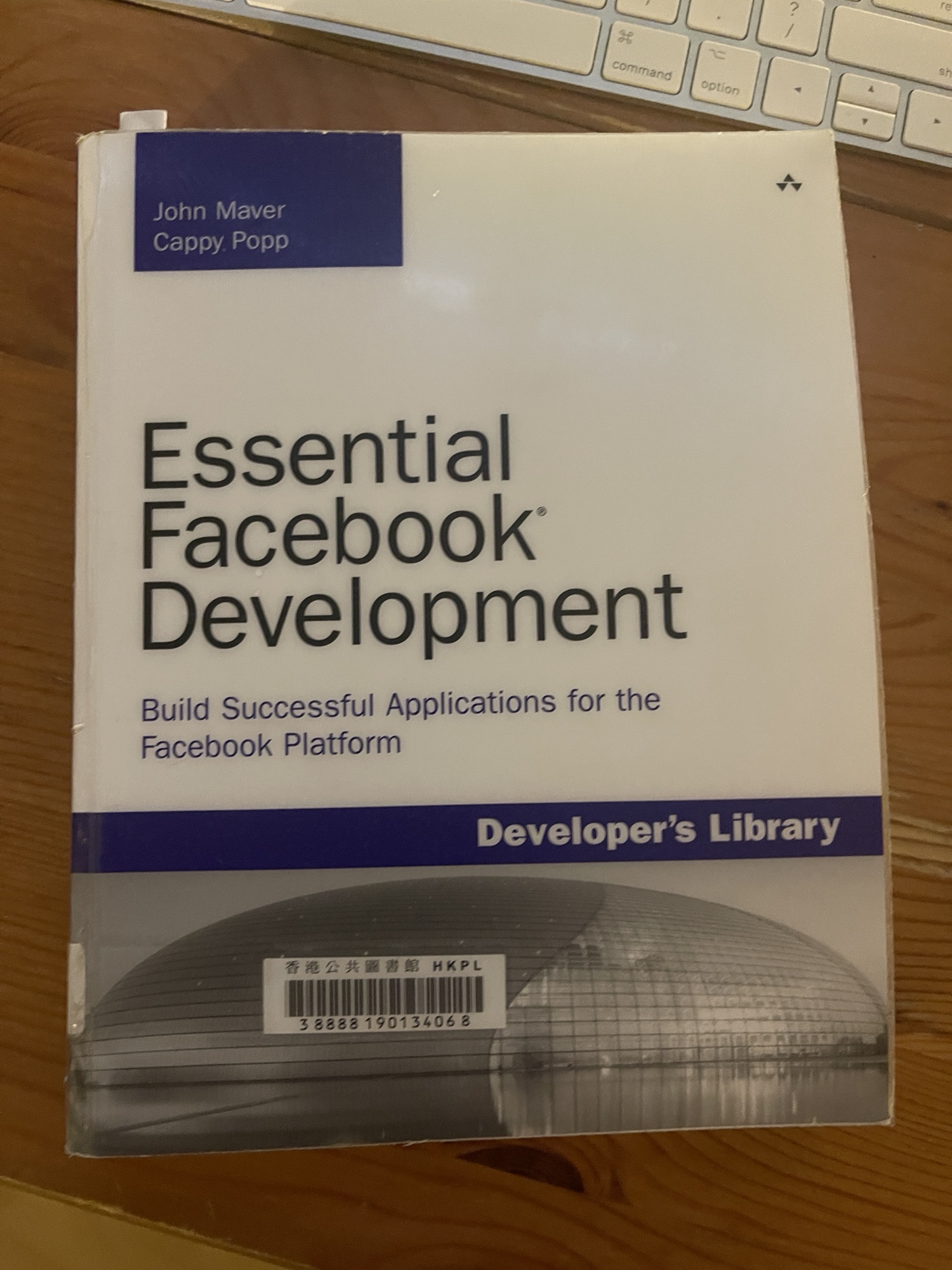
「代碼脫亞入歐」這一概念可以從幾個方面來理解,特別是在香港的編程界與日本的明治維新之間進行比較。
1. 歷史背景
- 明治維新:日本在19世紀中期至20世紀初的這場運動,旨在現代化國家,學習西方的技術和制度,從而提升國際競爭力。
- 香港編程界:香港的科技和編程文化需要吸收更多的國際元素,特別是西方先進的技術和創新思維。
2. 技術與創新
- 學習與引進:香港的程序員可以借鑒日本在技術引進和創新方面的成功經驗,積極學習全球最新的編程語言、開發工具和框架。
- 開源文化:推動開源項目和社區的發展,學習如何在全球範圍內合作和交流。
3. 教育與培訓
- 強化教育體系:借鑒日本的教育模式,提升編程教育的質量,例如加強STEM(科學、技術、工程和數學)教育。
- 實踐與實驗:鼓勵學生參加實習和實驗項目,增強實際操作能力。
4. 政策支持
- 政府角色:香港政府可以效仿日本的政策,創造良好的創新環境,支持初創企業和技術發展。
- 資金與投資:提供資助和資源,吸引更多國際企業和人才。
5. 文化與思維轉變
- 全球視野:推動編程文化向國際化轉型,培養開放和包容的心態。
- 創新思維:鼓勵創新和實驗,推動跨界合作,促進多元化的思維方式。
總結來說,「代碼脫亞入歐」不僅是技術上的轉型,更是一種思維方式和文化的變革。香港的編程界若能學習日本明治維新的經驗,將能在全球化的浪潮中更好地立足與發展。
This will select value in hex format, beware the bit width, otherwise it wrap to zero
SELECT SEQUENCE, RAWTOHEX(CAST(pc AS BINARY(8))), line FROM QUANTR ORDER BY SEQUENCE
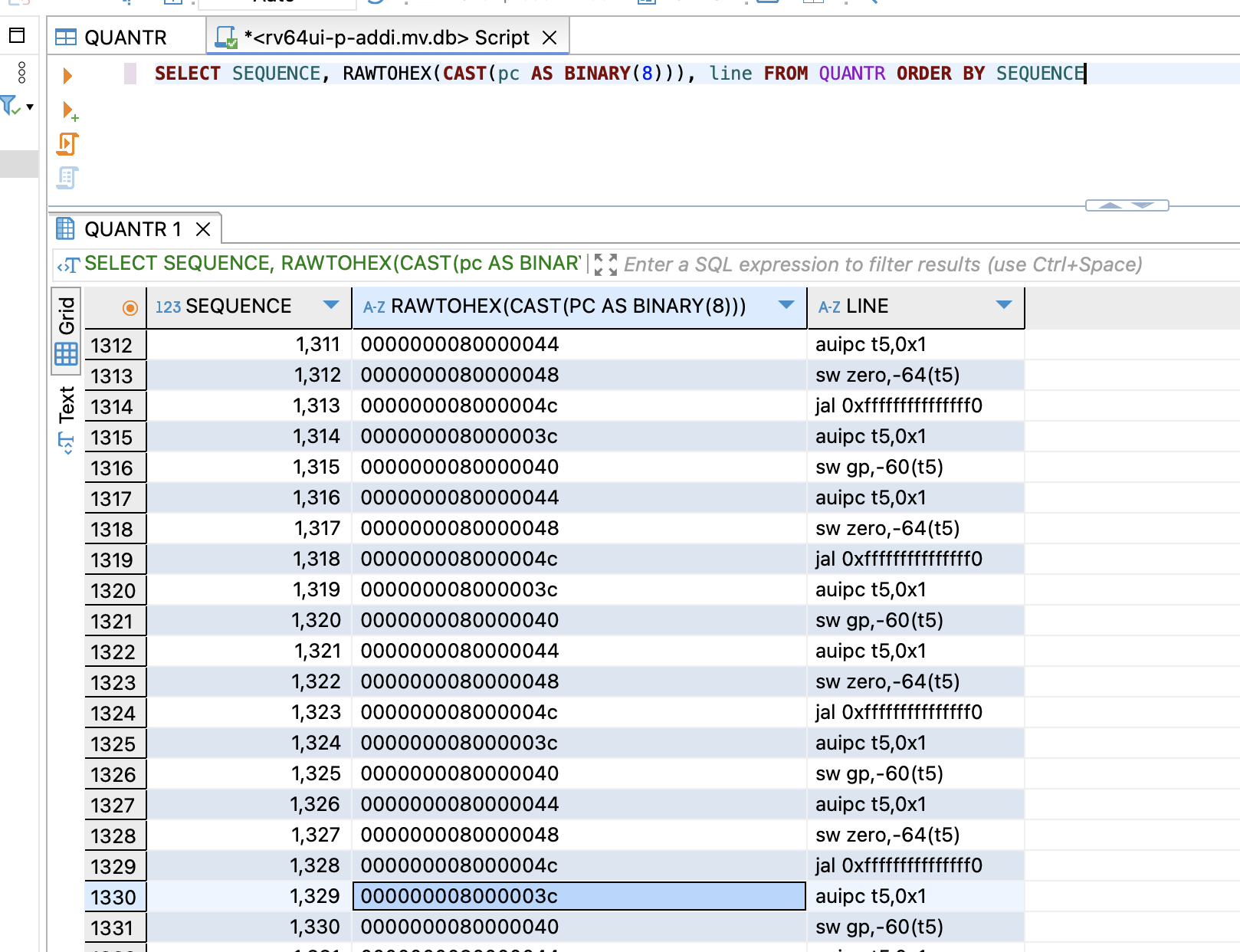
1. 時間係亂序執行,否則佛教不能自圓其說。只要亂序執行講得通,佛教整套哲學體系就會自己渝合。而家佛教哲學有幾個不能接合嘅地方,第一就係講所有野係空,但又因緣生。第二就係佛教嘅神通如果係真,根本容唔落係自己套哲學體系裏面。就算講得最清楚嘅龍樹,都只不過係話當「果」出現時,「因」先會被確定。如果時間係線性,呢單野根本完全唔合理,但如果時間就亂序執行,咁就好正確。因為係呢一對事物「因」+「果」佢地嘅時間當然係順序執行,但跳出左呢一對事物,佢地嘅時間線就唔係,所以係佢地時間線黎講果要出現左,因先可以成立。企左出去佢地嘅時間線,其實係果先出現,再到因。我再舉多個你地可以睇到嘅例子。int x; x=123. 呢兩句code,你地用verilator synthesis出logic gate,係呢兩句code嘅時間線上先有int x,先可以有x=123. 但其實係int x之前,係另一條時間線,x已經存在,所以果出現時,姐係int x出現時,佢個因x先被確立。乎合龍樹所講嘅野。古人因為科技所限,無法舉證。
2. 時間係唔係線性,而亂序執行唔會阻擋世情嘅出現。我地感受到時間係線性,因為只係我地要靠時間去圍持呢個身體同識。舉個實制例子,CPU係亂序執行,但被執行嘅program會以為自己嘅時間線係線性,但其實唔係。只係CPU唔會張下一句指令嘅執行結果話比上一句嘅指令知,最終令到program以為自己條時間線有如河流一樣線性。
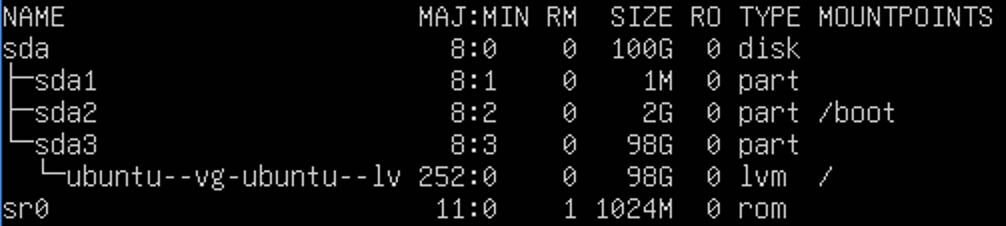
parted
resizepart 3
-0
quit
pvs
pvresize /dev/sda3
lvextend -l+100%FREE /dev/ubuntu-vg/ubuntu-lv
resize2fs /dev/ubuntu-vg/ubuntu-lv
WARNING: Retrying (Retry(total=4, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(SSLCertVerificationError(1, ‘[SSL: CERTIFICATE_VERIFY_FAILED] certificate verify failed: unable to get local issuer certificate (_ssl.c:992)’))’: /simple/matplotlib/
python -m pip install something --trusted-host pypi.python.org --trusted-host files.pythonhosted.org --trusted-host pypi.org
ls -tr|head -50|while read a; do echo $a; rm -fr $a/node_modules; done
https://www.accu.co.uk/p/117-iso-metric-thread-dimensions
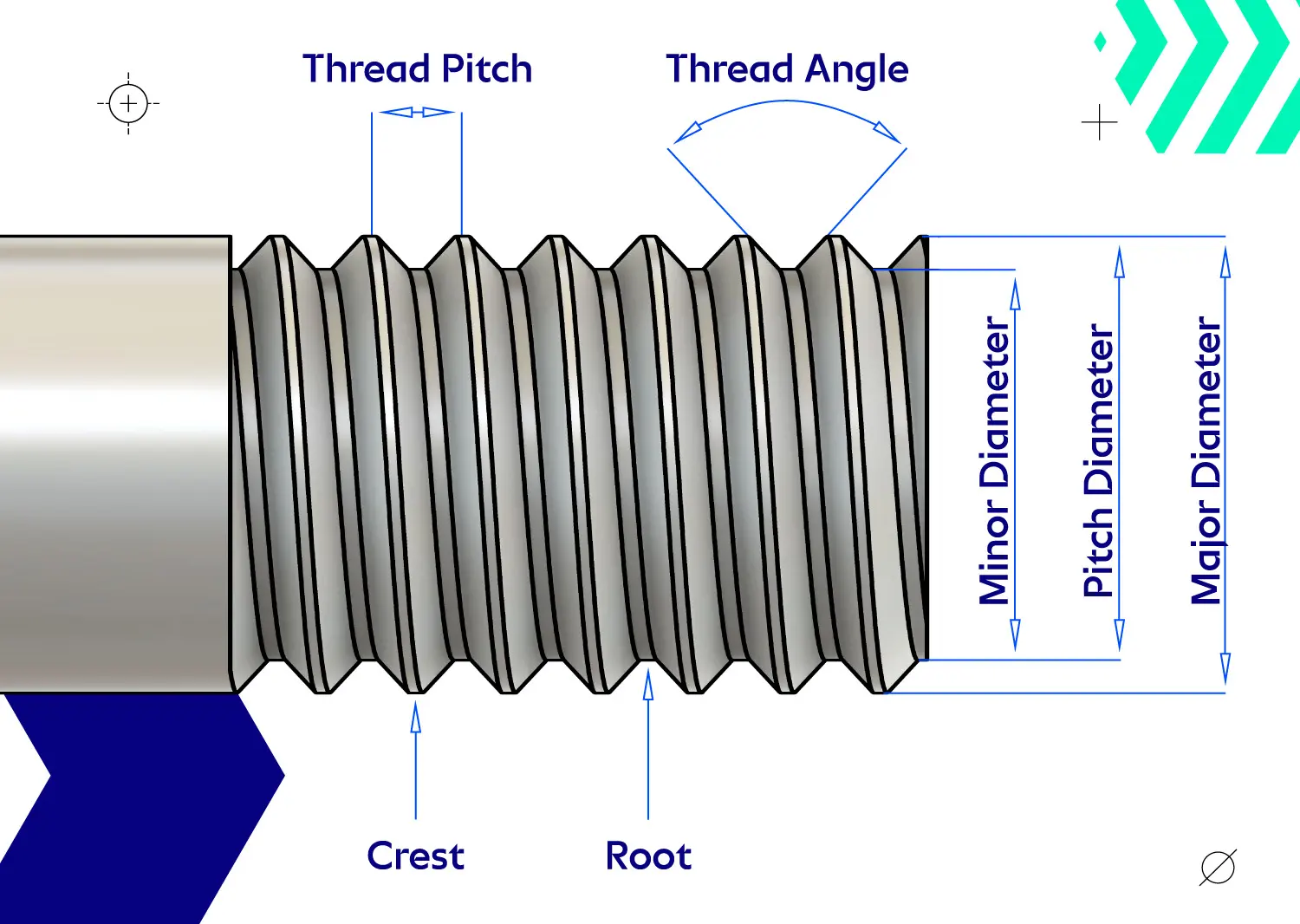
Bolt Sizes Chart & Metric Bolt Dimensions
THREAD SIZE | MAJOR DIAMETER (MM) | MINOR DIAMETER (MM) | THREAD PITCH (MM) | PITCH DIAMETER (MM) | TAPPING DRILL DIAMETER (MM) | CLEARANCE HOLE DIAMETER (MM) |
---|---|---|---|---|---|---|
M1 | 1.0 | 0.729 | 0.25 | 0.838 | 0.75 | 1.3 |
M1.1 | 1.1 | 0.829 | 0.25 | 0.938 | 0.85 | 1.4 |
M1.2 | 1.2 | 0.929 | 0.25 | 1.038 | 0.95 | 1.5 |
M1.4 | 1.4 | 1.075 | 0.30 | 1.205 | 1.10 | 1.8 |
M1.6 | 1.6 | 1.221 | 0.35 | 1.373 | 1.25 | 2.0 |
M1.8 | 1.8 | 1.421 | 0.35 | 1.573 | 1.45 | 2.3 |
M2 | 2.0 | 1.567 | 0.40 | 1.740 | 1.60 | 2.6 |
M2.2 | 2.2 | 1.713 | 0.45 | 1.908 | 1.75 | 2.9 |
M2.5 | 2.5 | 2.013 | 0.45 | 2.208 | 2.05 | 3.1 |
M3 | 3.0 | 2.459 | 0.50 | 2.675 | 2.50 | 3.6 |
M3.5 | 3.5 | 2.850 | 0.60 | 3.110 | 2.90 | 4.2 |
M4 | 4.0 | 3.242 | 0.70 | 3.545 | 3.30 | 4.8 |
M4.5 | 4.5 | 3.688 | 0.75 | 4.013 | 3.80 | 5.3 |
M5 | 5.0 | 4.134 | 0.80 | 4.480 | 4.20 | 5.8 |
M6 | 6.0 | 4.917 | 1.00 | 5.350 | 5.00 | 7.0 |
M7 | 7.0 | 5.917 | 1.00 | 6.350 | 6.00 | 8.0 |
M8 | 8.0 | 6.647 | 1.25 | 7.188 | 6.80 | 10.0 |
M9 | 9.0 | 7.647 | 1.25 | 8.188 | 7.80 | 11.0 |
M10 | 10.0 | 8.376 | 1.50 | 9.026 | 8.50 | 12.0 |
M11 | 11.0 | 9.376 | 1.50 | 10.026 | 9.50 | 13.5 |
M12 | 12.0 | 10.106 | 1.75 | 10.863 | 10.20 | 15.0 |
M14 | 14.0 | 11.835 | 2.00 | 12.701 | 12.00 | 17.0 |
M16 | 16.0 | 13.835 | 2.00 | 14.701 | 14.00 | 19.0 |
M18 | 18.0 | 15.394 | 2.50 | 16.376 | 15.50 | 22.0 |
M20 | 20.0 | 17.294 | 2.50 | 18.376 | 17.50 | 24.0 |
M22 | 22.0 | 19.294 | 2.50 | 20.376 | 19.50 | 26.0 |
M24 | 24.0 | 20.752 | 3.00 | 22.051 | 21.00 | 28.0 |
M27 | 27.0 | 23.752 | 3.00 | 25.051 | 24.00 | 33.0 |
M30 | 30.0 | 26.211 | 3.50 | 27.727 | 26.50 | 35.0 |
M33 | 33.0 | 29.211 | 3.50 | 30.727 | 29.50 | 38 |
M36 | 36.0 | 31.670 | 4.00 | 33.402 | 32.00 | 41 |
M39 | 39.0 | 34.670 | 4.00 | 36.402 | 35.00 | 44 |
M42 | 42.0 | 37.129 | 4.50 | 39.077 | 37.50 | 47 |
M45 | 45.0 | 40.129 | 4.50 | 42.077 | 40.50 | 50 |
M48 | 48.0 | 42.857 | 5.00 | 44.752 | 43.00 | 53 |
M52 | 52.0 | 46.587 | 5.00 | 48.752 | 47.00 | 57 |
M56 | 56.0 | 50.046 | 5.50 | 52.428 | 50.50 | 61 |
M60 | 60.0 | 54.046 | 5.50 | 56.428 | 54.50 | 65 |
M64 | 64.0 | 57.505 | 6.00 | 60.103 | 58.00 | 69 |
M68 | 68.0 | 61.505 | 6.00 | 64.103 | 62.00 | 73 |
165 23.534334 192.168.10.115 218.255.142.226 DNS 70 Standard query 0x1644 A quantr.com
0000 b4 75 0e 66 6c f2 78 4f 43 9c 8c 15 08 00 45 00 .u.fl.xOC.....E.
0010 00 38 67 33 00 00 40 11 de 84 c0 a8 0a 73 da ff [email protected]..
0020 8e e2 ff e6 00 35 00 24 86 8b 16 44 01 00 00 01 .....5.$...D....
0030 00 00 00 00 00 00 06 71 75 61 6e 74 72 03 63 6f .......quantr.co
0040 6d 00 00 01 00 01 m.....
166 23.538999 218.255.142.226 192.168.10.115 DNS 86 Standard query response 0x1644 A quantr.com A 218.255.142.226
0000 78 4f 43 9c 8c 15 b4 75 0e 66 6c f2 08 00 45 00 xOC....u.fl...E.
0010 00 48 3a cf 00 00 37 11 13 d9 da ff 8e e2 c0 a8 .H:...7.........
0020 0a 73 00 35 ff e6 00 34 86 f3 16 44 85 00 00 01 .s.5...4...D....
0030 00 01 00 00 00 00 06 71 75 61 6e 74 72 03 63 6f .......quantr.co
0040 6d 00 00 01 00 01 c0 0c 00 01 00 01 00 01 51 80 m.............Q.
0050 00 04 da ff 8e e2 ......