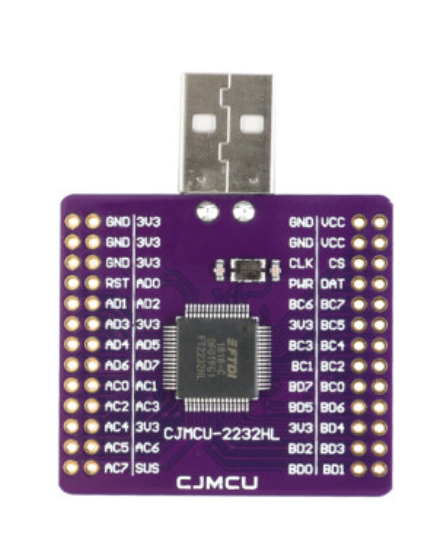
Bought from here . Example is here
#!/usr/bin/env python3
from os import environ
from pyftdi.jtag import JtagEngine, JtagTool
from pyftdi.bits import BitSequence
JTAG_INSTR = {
'SAMPLE' : BitSequence('0000000101', msb=True, length=10),
'IDCODE' : BitSequence('0000000110', msb=True, length=10),
'USERCODE': BitSequence('0000000111', msb=True, length=10),
'BYPASS' : BitSequence('1111111111', msb=True, length=10)}
url = environ.get('FTDI_DEVICE', 'ftdi://ftdi:2232h/1')
jtag = JtagEngine(trst=True, frequency=3E6)
jtag.configure(url)
jtag.reset()
def read_idcode(jtag):
instr = JTAG_INSTR['IDCODE']
jtag.reset()
jtag.write_ir(instr)
idcode = jtag.read_dr(32)
jtag.go_idle()
print(" IDCODE: 0x%08x" % int(idcode))
def read_usercode(jtag):
instr = JTAG_INSTR['USERCODE']
jtag.write_ir(instr)
idcode = jtag.read_dr(32)
jtag.go_idle()
print("USERCODE: 0x%08x" % int(idcode))
if __name__ == '__main__':
read_idcode(jtag)
read_usercode(jtag)
del jtag
# altera_ep4ce6e22: 0x020f10dd
# altera_ep4ce15e22: 0x020f30dd
Read PWM
#!/usr/bin/env python3
from pyftdi.jtag import JtagEngine
from pyftdi.bits import BitSequence
import time
FTDI_BOARD = 'ftdi://ftdi:2232h/1'
SPEED = 5
TCK_FREQ = 1e6
MAX_WIDTH = 255
def read_pwm():
jtag.write_ir(RD_PWM)
pwm = jtag.read_dr(1)
print("PWM: 0x%x" % int(pwm))
# Define Instructions
IDCODE = BitSequence('00000100', msb=True, length=8)
WR_WIDTH = BitSequence('00000101', msb=True, length=8)
RD_PWM = BitSequence('00000110', msb=True, length=8)
# Initialize
jtag = JtagEngine(frequency=TCK_FREQ)
jtag.configure(FTDI_BOARD)
jtag.reset() # Test Logic Reset (5 times of TMS=1)
# To Shift-IR, Exit1-IR and finally move to UpdateIR
jtag.write_ir(IDCODE)
# Move to Shift-DR, repeat Shift-DR, wait 15ms, and move to Test-Logic Reset
idcode = jtag.read_dr(32)
print("IDCODE (reset): 0x%x" % int(idcode))
width = 0
dir = 1
while True:
jtag.write_ir(WR_WIDTH)
jtag.write_dr(BitSequence(width, msb=False, length=8))
width += dir * SPEED
if width >= MAX_WIDTH:
read_pwm()
dir = -1
width = MAX_WIDTH
elif width <= 0:
read_pwm()
dir = 1
width = 0
time.sleep(0.001)
Pins reference : https://ftdichip.com/wp-content/uploads/2020/07/AN_184-FTDI-Device-Input-Output-Pin-States.pdf
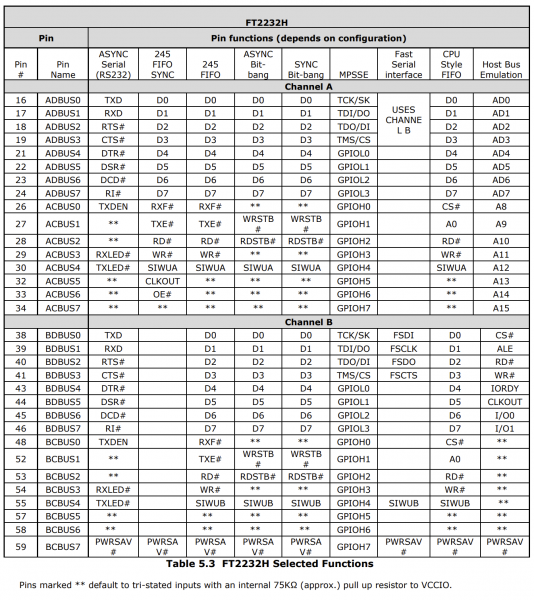