A little bit better – Showing the MBR
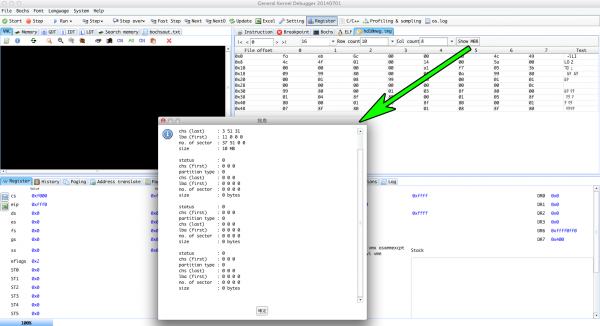
Here is the java code to read MBR out:
package com.peterswing.mbr; import java.io.FileInputStream; import java.io.InputStream; import org.apache.commons.io.IOUtils; import com.peterswing.CommonLib; public class ReadMBR { public static void main(String[] args) { try { InputStream in = new FileInputStream("/Users/peter/workspace/GKD/vm/dlxlinux/hd10meg.img"); in.skip(0x1be); for (int x = 0; x < 4; x++) { byte partition[] = new byte[16]; IOUtils.read(in, partition, 0, 16); printPartitionTable(partition); } IOUtils.closeQuietly(in); } catch (Exception ex) { ex.printStackTrace(); } } public static String getPartitionTable(byte partition[]) { String s = ""; s += "status : " + partition[0] + "\n"; s += "chs (first) : " + hex(partition[1]) + " " + hex(partition[2]) + " " + hex(partition[3]) + "\n"; s += "partition type : " + hex(partition[4]) + "\n"; s += "chs (last) : " + hex(partition[5]) + " " + hex(partition[6]) + " " + hex(partition[7]) + "\n"; s += "lba (first) : " + hex(partition[8]) + " " + hex(partition[9]) + " " + hex(partition[10]) + " " + hex(partition[11]) + "\n"; s += "no. of sector : " + hex(partition[12]) + " " + hex(partition[13]) + " " + hex(partition[14]) + " " + hex(partition[15]) + "\n"; s += "size : " + CommonLib.convertFilesize(CommonLib.getInt(new byte[] { partition[12], partition[13], partition[14], partition[15] }, 0) * 512) + "\n"; return s; } public static void printPartitionTable(byte partition[]) { System.out.println(getPartitionTable(partition)); } public static String hex(int x) { return Integer.toHexString(x & 0xff); } }